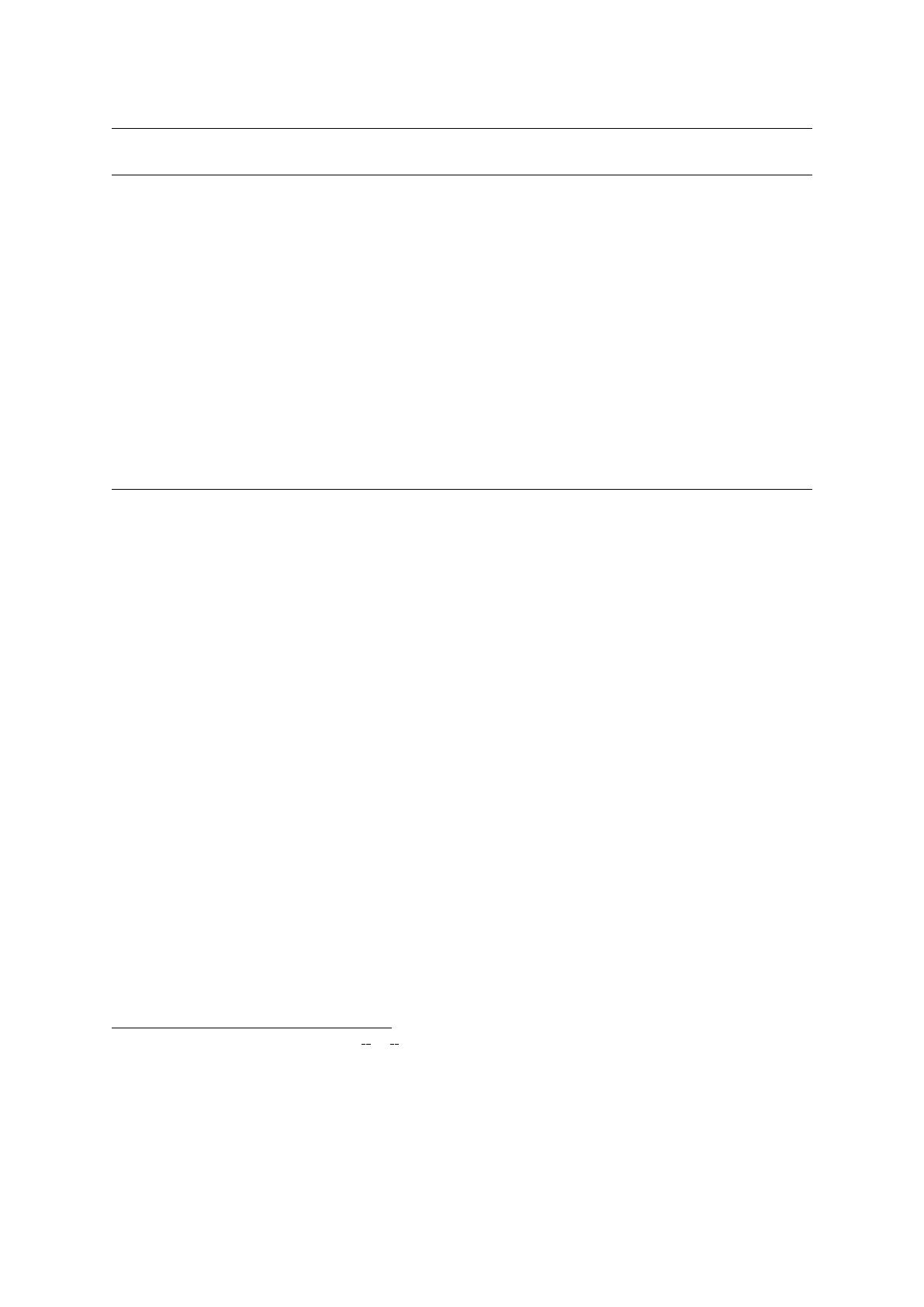
IN101 - TD07 ´
Enonc´
e
Instructions g ´
en´
erales
•En cas de probl`
emes, demandez de l’aide! Mais n’oubliez pas de vous r´
ef´
erer, en premier lieu, au diapos-
itives du cours magistral et aux r ´
ef´
erences bibliographiques. L’index disponible en ligne vous aidera `
a lier
concepts abord´
es et suports de cours.
•Utilisez l’´
editeur de texte de votre choix pour les exercices. En cas d’h´
esitation, privil ´
egiez gedit.
•Si ce n’est pas encore le cas, cr´
eez un dossier IN101/.
•Dans ce dossier IN101/, cr´
eez un nouveau sous-dossier pour la s´
eance de TD, que vous nommerez TD07/.
•Pour les questions du type : ”Write, in natural language, an algorithm that. . . ”, ouvrez un nouveau fichier
texte (d’extension ”.txt”) plutˆ
ot qu’un fichier python (d’extension ”.py”).
Exemple : helloworld.txt
•Documentez et commentez pr´
ecis´
ement votre code : d ´
ecrivez l’objectif g´
en´
eral de l’algorithme, expliquez
les points-clefs de son impl´
ementation, d´
etaillez les conditions normales d’ ´
ex´
ecution du script (c.- `
a-d. le
fichier ”.py”) et exposez les cas extrˆ
emes et les erreurs pr´
evus.
Everyone
1 Hashing
A hash table is a collection of items which are stored in such a way as to make it easy to find
them later. Each position of the hash table, often called a slot, can hold an item and is named
by an integer value starting at 0. For example, we will have a slot named 0, a slot named 1, a
slot named 2, and so on. Initially, the hash table contains no items so every slot is empty.
2 HashTable creation
We can implement a hash table by using a list with each element initialized to the special
Python value None. A second list can be used to store the actual data.
Aims: Implement a class for hashtables. Implement functions with pre-defined specifications.
As in the CM, implement a HashTable class in the HashTable.py module, and implement
the member functions below1. For Q6,Q7, and Q8, we recommend that you draw the different
steps of the function (with links between nodes, as in the CM) on a piece of paper before
implementing it in Python.
You can find the departure code at
http://perso.ensta-paristech.fr/~paun/ENSTA_IN101/hash_table_td.py.
1Note that calling the length function len allows you to call len(H) on a HashTable H.
1