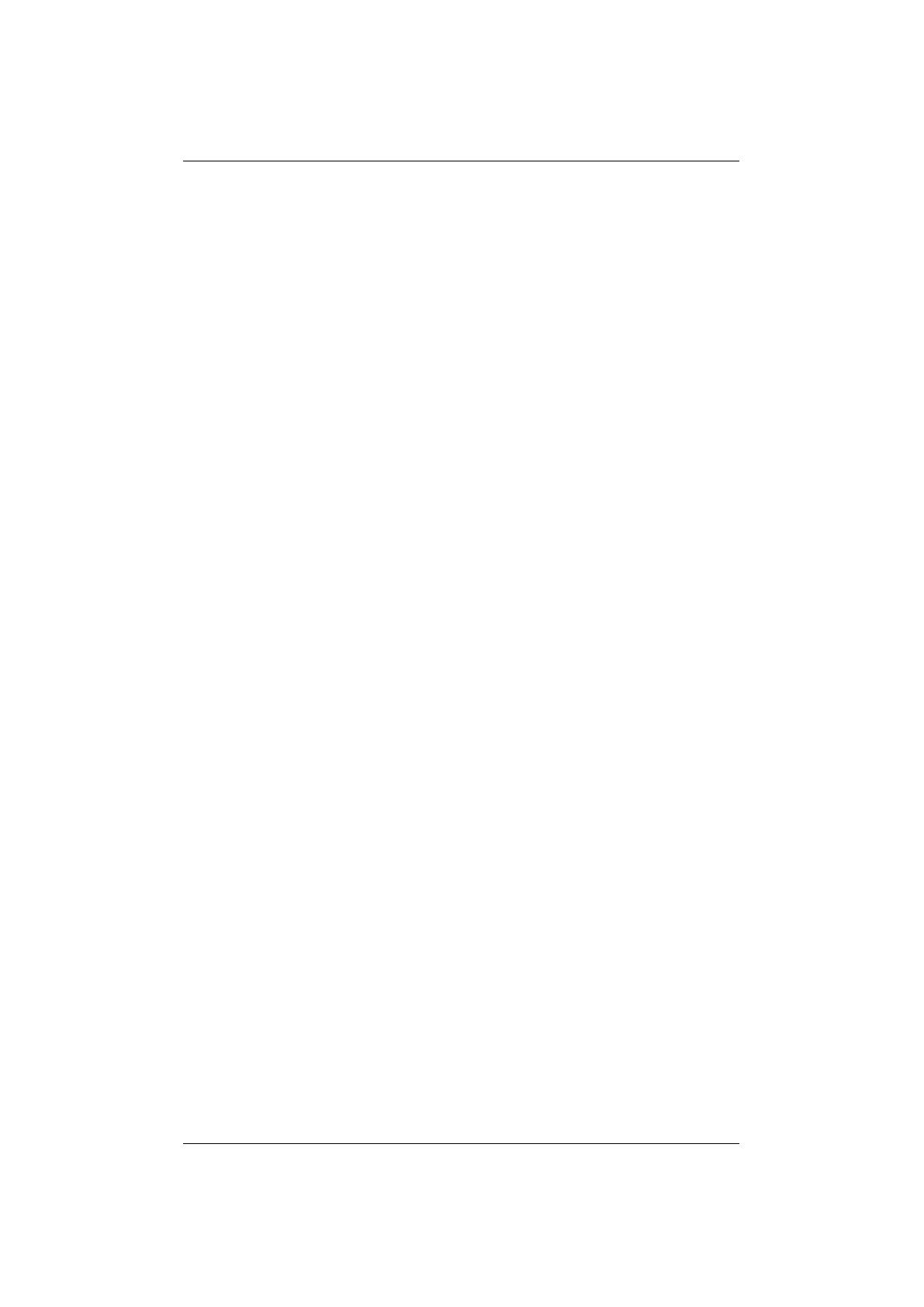
TP 1 : Introduction à la programmation graphique en Java
1er février 2017
1 Programmation d’une montre digitale
On voudrais développer une class abstraite Montre qui implante une
montre digitale. La classe montre utilise une autre classe abstraite Afficheur
qui permet d’afficher l’heure. Cette classe offre deux méthodes abstraites
d’affichage de l’heure. La première méthode acceptent en entrée trois entiers
qui représentent respectivement, les heures, les minutes et les secondes. La
deuxième méthode prends en entrée une chaîne de caractères qui représente
l’heure à afficher.
Différents types d’afficheurs peuvent être imaginés alors : en mode texte,
en mode graphique, etc. Pour réaliser une classe concrète qui implante une
montre on doit alors spécifier une classe concrète d’affichage qui hérite de
la classe Afficheur et une classe concrète de montre qui hérite de la classe
Montre
— Définir la classe abstraite Afficheur
public abstract class Affich eur {
public abstract void afficher(int h , int m , int s );
public abst ract void aff icher ( String s );
/* m ethode p our af fic he r l ’ he ure */
}
— Définir la classe abstraite Montre
import java . text .*;
abstract class Montre extends Thread {
private int s ,m , h;
protec ted Affi cheur affiche ;
protec ted boole an am ;
private boolean mode24;
private boolean on ;
public Montre (){
this. s =0;
this. m =0;
this. h =0;
}
1