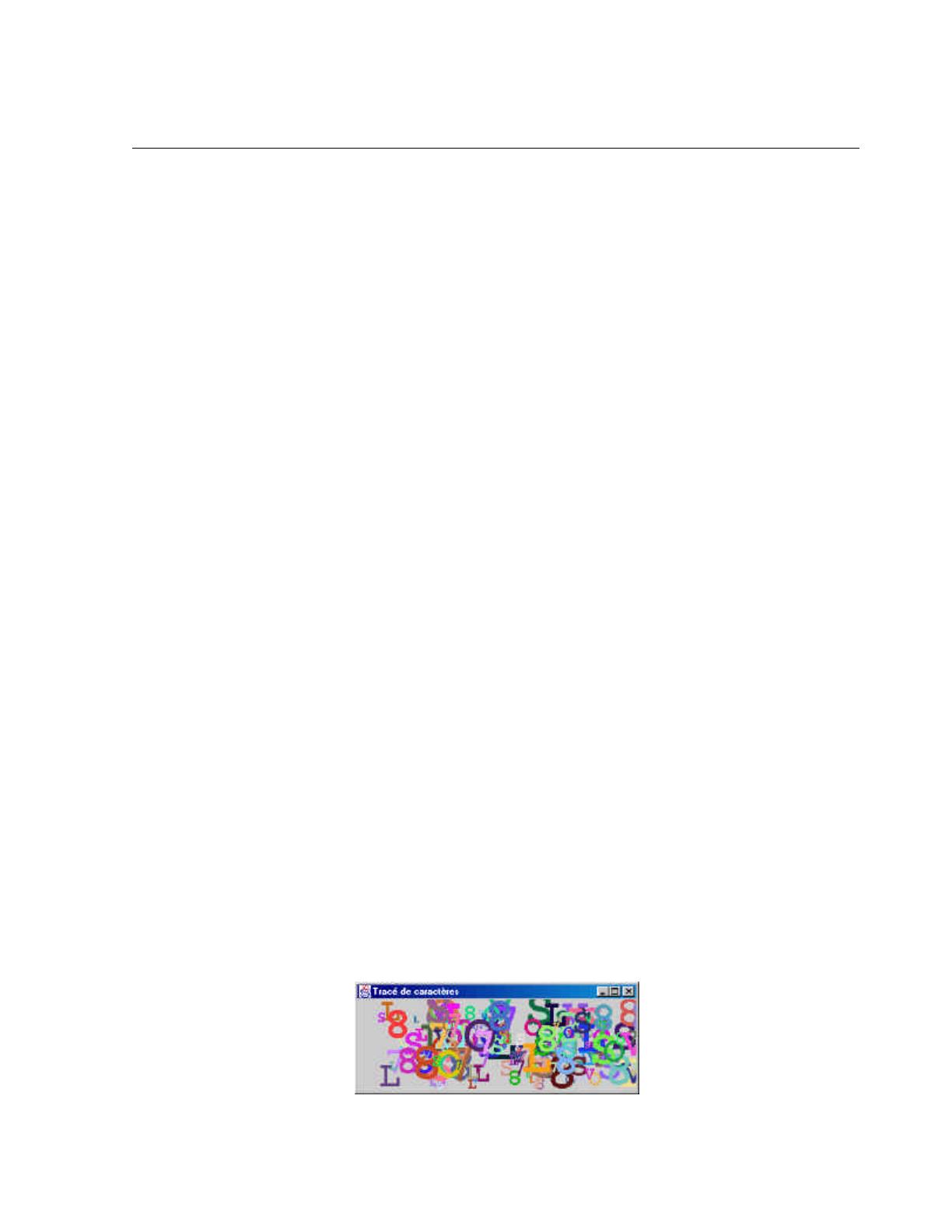
COPYRIGHT 2001 LES ÉDITIONS REYNALD GOULET INC. TOUTE REPRODUCTION INTERDITE.
11.12 Écrivez un programme qui dessine des caractères dans des tailles de polices et des couleurs différentes.
RÉP.:
1// Solution de l’exercice 11.12.
2// Dessin.java
3// Ce programme dessine des caractères au hasard.
4// Note: couvrir, re-dimensionner ou re-démarrer le programme de manière
5// répétitive pour voir le tracé de plusieurs caractères.
6import javax.swing.*;
7import java.awt.*;
8import java.awt.event.*;
9
10 public class Dessin extends JFrame {
11 private final int DELAI = 4000000;
12
13 public Dessin()
14 {
15 super( "Tracé de caractères" );
16 setSize( 380, 150 );
17 show();
18 }
19
20 public void paint( Graphics g )
21 {
22 int taillePolice = ( int ) ( 10 + Math.random() * 63 );
23 int x = ( int ) ( 30 + Math.random() * 341 );
24 int y = ( int ) ( 50 + Math.random() * 95 );
25 char lettres[] = { 'V', 'O', 'L', 'S', '8', '7' };
26 Font f = new Font( "Monospaced", Font.BOLD, taillePolice );
27
28 g.setColor( new Color( ( float ) Math.random(),
29 ( float ) Math.random(),
30 ( float ) Math.random() ) );
31 g.setFont( f );
32 g.drawChars( lettres, ( int ) ( Math.random() * 6 ), 1, x, y );
33
34 for ( int h = 1; h < DELAI; h++ ) ; // Ralentir l’affichage.
35 repaint();
36 }
37
38 public static void main( String args[] )
39 {
40 Dessin app = new Dessin();
41
42 app.addWindowListener(
43 new WindowAdapter() {
44 public void windowClosing( WindowEvent e )
45 {
46 System.exit( 0 );
47 }
48 }
49 );
50 }
51 }
11.13 Écrivez un programme qui dessine une grille de 8 sur 8. Employez la méthode drawLine.
RÉP.: