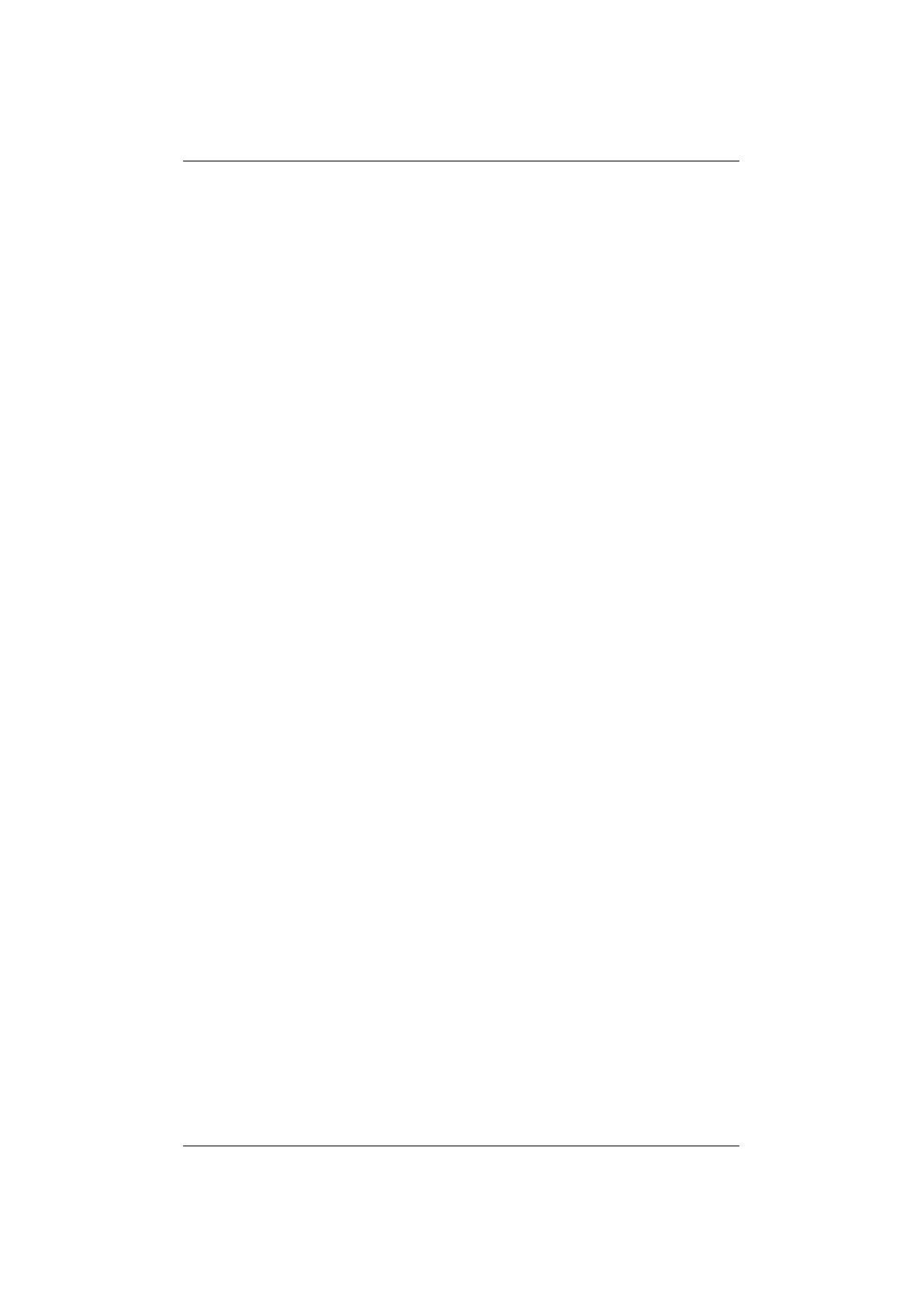
class ClientServeurUDP
import java.net.*;
import java.io.*;
public abstract class ClientServeurUDP implements ClientServeur
{ protected DatagramSocket socket;
protected InetAddress adresseIPDistante;
protected int portDistant;
public void envoyerMessage(String message) throws IOException
{// construction du paquet surdimensionné
DatagramPacket packet = new DatagramPacket(message.getBytes(),message.length(),adresseIPDistante,portDistant);
socket.send(packet);
}
// méthode recevoirMessage du serveur
public String recevoirMessage()throws IOException
{ byte buffer[]=new byte[16000];
DatagramPacket packet = new DatagramPacket(buffer,buffer.length);// préparation du packet vide
socket.receive(packet);
this.portDistant=packet.getPort();
this.adresseIPDistante=packet.getAddress();
return(new String(packet.getData()).trim());
}
}//fin de la classe ClientServeurUDP
class TableTemp
import java.util.*;
public class TableTemp extends Observable {
private static int mid; /* messge id*/
private Hashtable<Integer,String> tab_m; // table des messges
private Hashtable<Integer,STTimer> tab_t; // table de temporisateurs
private int timeout; /* duree du temporisateurs */
public TableTemp(int timeout) throws Exception {
if (timeout > 1000){ // au moins une second
2