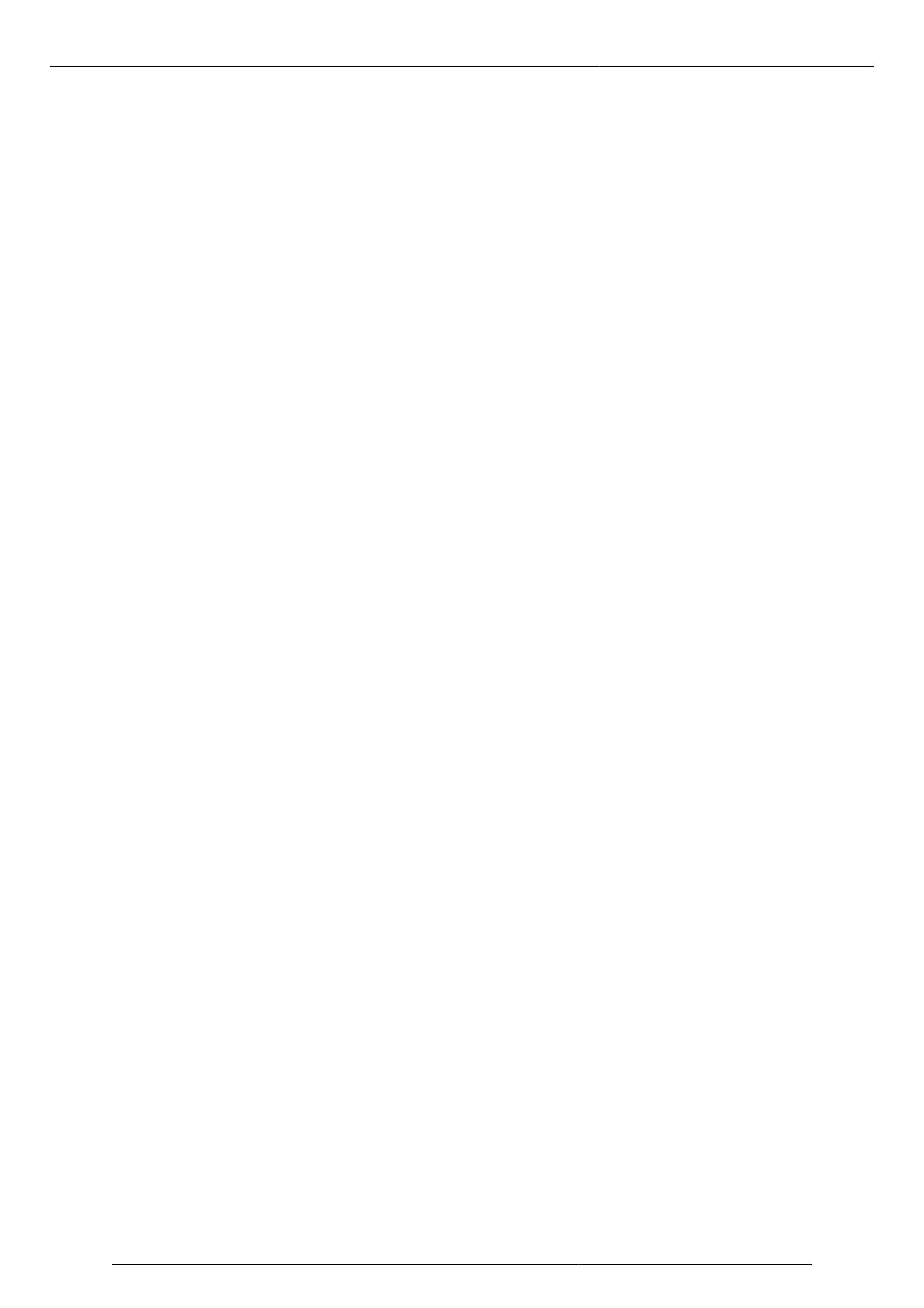
Spring Framework Reference Documentation
3.2.18.RELEASE Spring Framework v
Instantiation using an instance factory method ........................................... 41
5.4. Dependencies ................................................................................................... 42
Dependency injection ....................................................................................... 42
Constructor-based dependency injection .................................................... 43
Setter-based dependency injection ............................................................ 45
Dependency resolution process ................................................................. 46
Examples of dependency injection ............................................................. 47
Dependencies and configuration in detail ........................................................... 49
Straight values (primitives, Strings, and so on) ....................................... 49
References to other beans (collaborators) .................................................. 50
Inner beans .............................................................................................. 51
Collections ............................................................................................... 51
Null and empty string values ..................................................................... 54
XML shortcut with the p-namespace .......................................................... 54
XML shortcut with the c-namespace .......................................................... 55
Compound property names ....................................................................... 56
Using depends-on .......................................................................................... 57
Lazy-initialized beans ....................................................................................... 57
Autowiring collaborators .................................................................................... 58
Limitations and disadvantages of autowiring ............................................... 59
Excluding a bean from autowiring .............................................................. 59
Method injection ............................................................................................... 60
Lookup method injection ........................................................................... 60
Arbitrary method replacement ................................................................... 62
5.5. Bean scopes ..................................................................................................... 63
The singleton scope ......................................................................................... 64
The prototype scope ......................................................................................... 64
Singleton beans with prototype-bean dependencies ............................................ 65
Request, session, and global session scopes .................................................... 66
Initial web configuration ............................................................................ 66
Request scope ......................................................................................... 66
Session scope .......................................................................................... 67
Global session scope ............................................................................... 67
Scoped beans as dependencies ................................................................ 67
Custom scopes ................................................................................................ 69
Creating a custom scope .......................................................................... 69
Using a custom scope .............................................................................. 70
5.6. Customizing the nature of a bean ....................................................................... 71
Lifecycle callbacks ............................................................................................ 71
Initialization callbacks ............................................................................... 72
Destruction callbacks ................................................................................ 72
Default initialization and destroy methods .................................................. 73
Combining lifecycle mechanisms ............................................................... 74
Startup and shutdown callbacks ................................................................ 75
Shutting down the Spring IoC container gracefully in non-web applications
................................................................................................................. 76
ApplicationContextAware and BeanNameAware ........................................ 77
Other Aware interfaces .................................................................................... 78
5.7. Bean definition inheritance ................................................................................. 79
5.8. Container Extension Points ................................................................................ 80