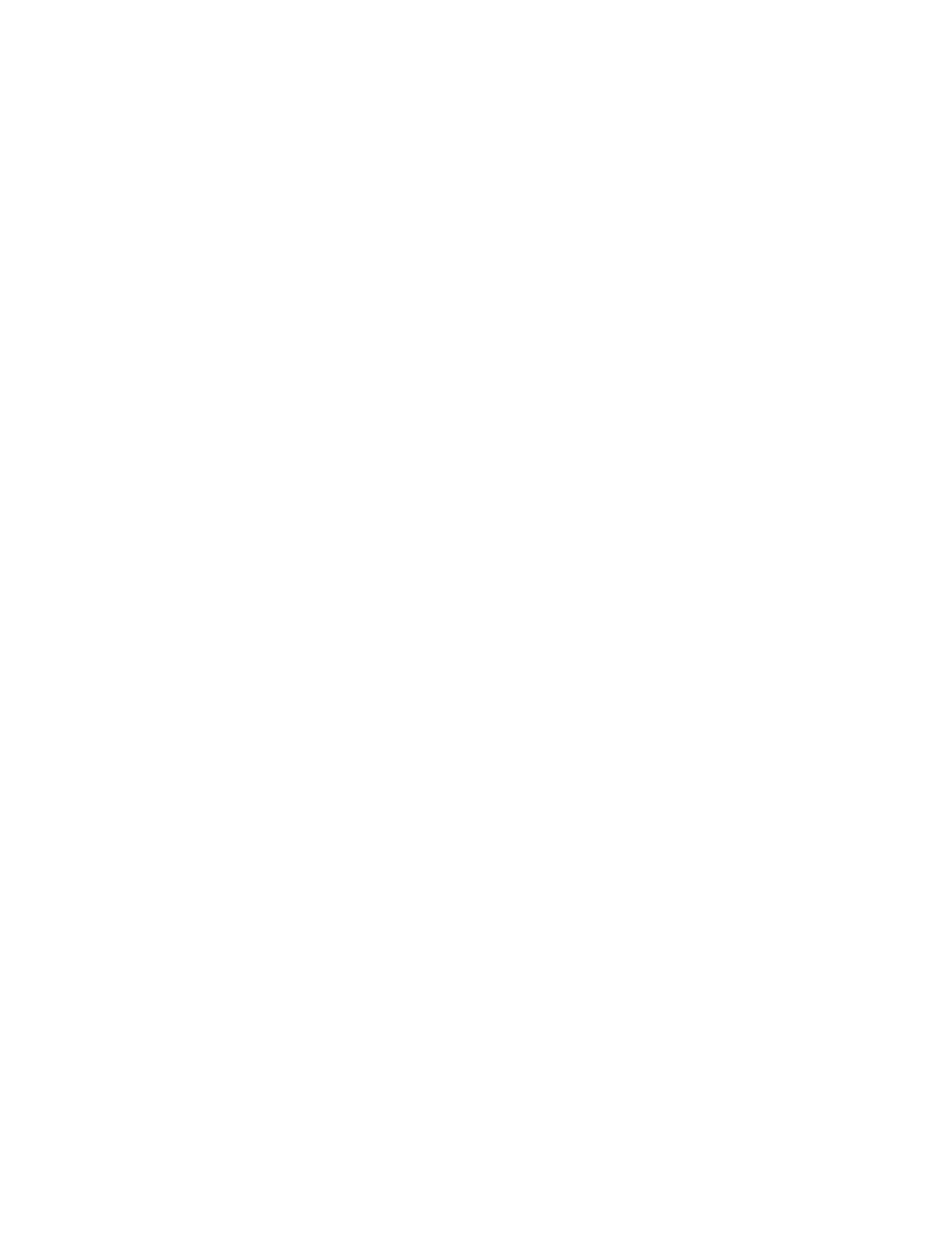
CONTENTS 5
9.3 Pattern matching on lists . . . . . . . . . . . . . . . . . . 294
9.4 List’s syntactic sugar . . . . . . . . . . . . . . . . . . . . . 296
9.5 Using ranges to construct lists . . . . . . . . . . . . . . . 297
9.6 Extracting portions of lists . . . . . . . . . . . . . . . . . 299
9.7 List comprehensions . . . . . . . . . . . . . . . . . . . . . 303
9.8 Spines and non-strict evaluation . . . . . . . . . . . . . 307
9.9 Transforming lists of values . . . . . . . . . . . . . . . . . 315
9.10 Filtering lists of values . . . . . . . . . . . . . . . . . . . . 321
9.11 Zippinglists...........................323
9.12 Chapter Exercises . . . . . . . . . . . . . . . . . . . . . . . 325
9.13 Definitions............................331
9.14 Answers..............................332
9.15 Follow-up resources . . . . . . . . . . . . . . . . . . . . . . 335
10 Folding lists 336
10.1 Folds................................337
10.2 Bringing you into the fold . . . . . . . . . . . . . . . . . . 337
10.3 Recursive patterns . . . . . . . . . . . . . . . . . . . . . . . 338
10.4 Foldright.............................339
10.5 Foldle..............................345
10.6 How to write fold functions . . . . . . . . . . . . . . . . . 353
10.7 Folding and evaluation . . . . . . . . . . . . . . . . . . . . 357
10.8 Summary ............................358
10.9 Scans ...............................359
10.10 Chapter Exercises . . . . . . . . . . . . . . . . . . . . . . . 362
10.11 Definitions............................367
10.12 Answers..............................368
10.13 Follow-up resources . . . . . . . . . . . . . . . . . . . . . . 374
11 Algebraic datatypes 375
11.1 Algebraic datatypes . . . . . . . . . . . . . . . . . . . . . . 376
11.2 Data declarations review . . . . . . . . . . . . . . . . . . . 376
11.3 Data and type constructors . . . . . . . . . . . . . . . . . 378
11.4 Data constructors and values . . . . . . . . . . . . . . . . 380
11.5 What’s a type and what’s data? . . . . . . . . . . . . . . . 384
11.6 Data constructor arities . . . . . . . . . . . . . . . . . . . 387
11.7 What makes these datatypes algebraic? . . . . . . . . . 389
11.8 Sumtypes ............................397
11.9 Producttypes..........................399
11.10 Normalform ..........................403
11.11 Constructing and deconstructing values . . . . . . . . . 406
11.12 Function type is exponential . . . . . . . . . . . . . . . . 419
11.13 Higher-kinded datatypes . . . . . . . . . . . . . . . . . . 423