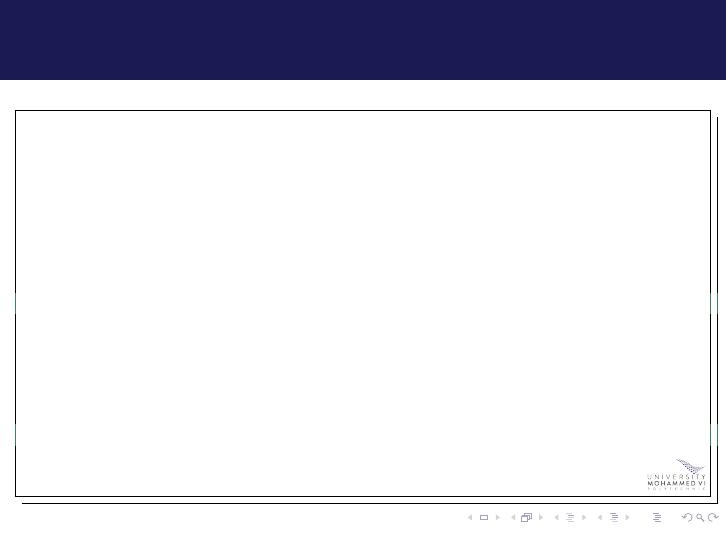
Map as Python Dictionary
Lines 1–15 / 19
1import sys
f i l e n a m e = s y s . ar gv [ 1 ]
3
f r e q = {}
5for piece in open ( f i l e n a m e ) . r ea d ( ) . l ow er () . s p l i t ( ) :
# o nl y c o n s i d e r a l p h a b e t i c c h a r a c t e r s w i t h i n t h i s p i e c e
7word = ’ ’ . j o i n ( c for cin piece i f c . i s a l p h a ( ) )
i f word : # r e q u i r e a t ⤦
Çl e a s t one a l p h a b e t i c c h a r a c t e r
9f r e q [ word ] = 1 + f r e q . get ( word , 0 )
11 max_word = ’ ’
max_count = 0
13 for (w, c ) in f r e q . i t e m s ( ) : # ( key , v a l u e ) t u p l e s ⤦
Çr e p r e s e n t ( word , c o unt )
i f c > max_count :
15 max_word = w
4 / 43