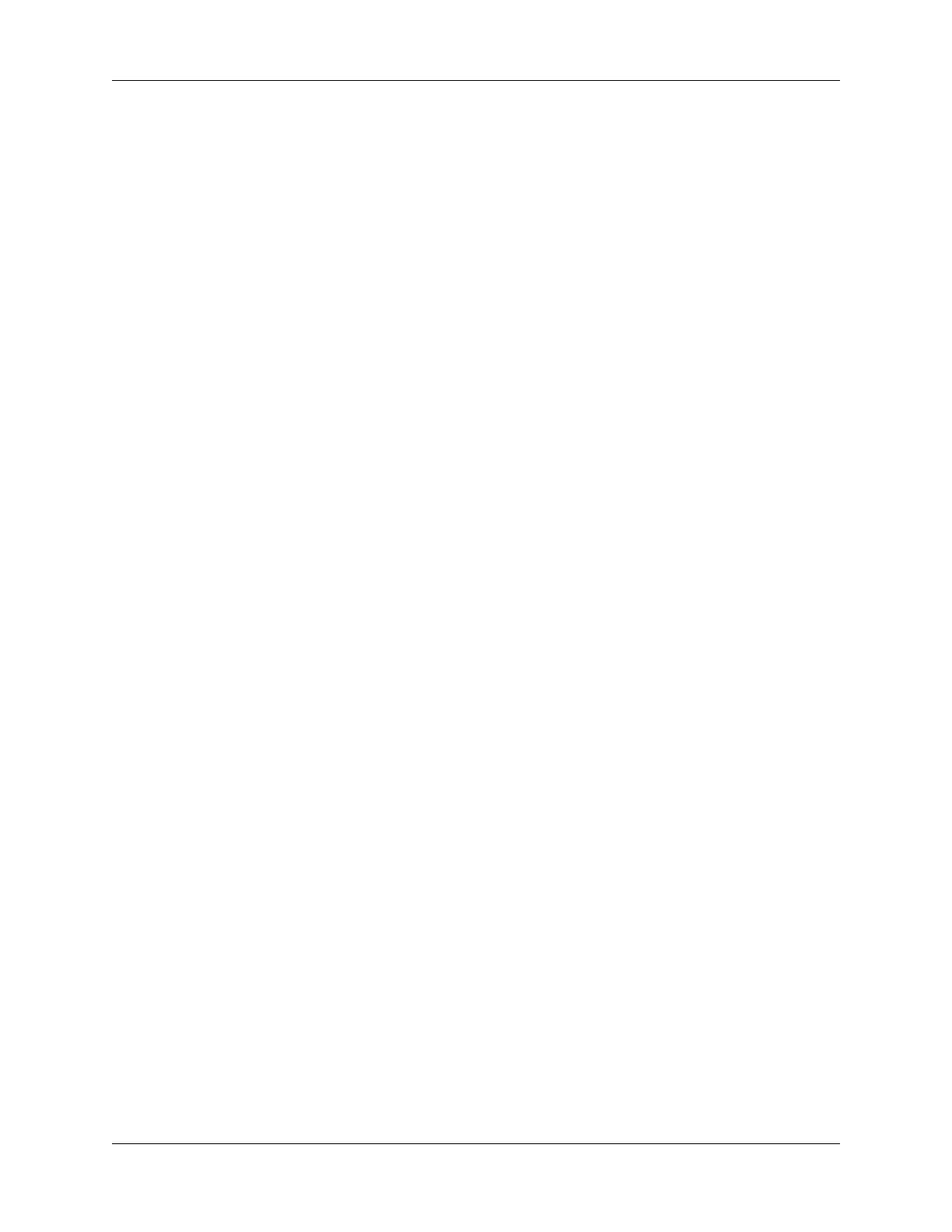
Machine Learning A-Z Q&A
Table of contents
1 Part 1 - Data Preprocessing 4
1.1 Importingthedataset ........................................ 4
1.2 MissingData ............................................. 4
1.3 CategoricalData ........................................... 5
1.4 Splitting the dataset into the Training set and Test set . . . . . . . . . . . . . . . . . . . . . . 5
1.5 FeatureScaling............................................ 5
2 Part 2 - Regression 6
2.1 SimpleLinearRegression ...................................... 6
2.1.1 Simple Linear Regression Intuition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.1.2 Simple Linear Regression in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.1.3 Simple Linear Regression in R . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
2.2 MultipleLinearRegression ..................................... 7
2.2.1 Multiple Linear Regression Intuition . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
2.2.2 Multiple Linear Regression in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
2.2.3 Multiple Linear Regression in R . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.3 PolynomialRegression........................................ 8
2.3.1 Polynomial Regression Intuition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 8
2.3.2 Polynomial Regression in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 9
2.3.3 PolynomialRegressioninR................................. 9
2.4 SVR .................................................. 9
2.4.1 SVRIntuition......................................... 9
2.4.2 SVRinPython........................................ 10
2.4.3 SVRinR ........................................... 10
2.5 DecisionTreeRegression....................................... 10
2.5.1 Decision Tree Regression Intuition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
2.5.2 Decision Tree Regression in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
2.5.3 Decision Tree Regression in R . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.6 RandomForestRegression...................................... 11
2.6.1 Random Forest Regression Intuition . . . . . . . . . . . . . . . . . . . . . . . . . . . . 11
2.6.2 Random Forest Regression in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
2.6.3 Random Forest Regression in R . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
2.7 Evaluating Regression Models Performance . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
3 Part 3 - Classification 14
3.1 LogisticRegression.......................................... 14
3.1.1 Logistic Regression Intuition . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
3.1.2 Logistic Regression in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
3.1.3 LogisticRegressioninR................................... 16
3.2 K-NearestNeighbors(K-NN) .................................... 17
3.2.1 K-NNIntuition........................................ 17
3.2.2 K-NNinPython ....................................... 17
3.2.3 K-NNinR .......................................... 17
3.3 SupportVectorMachine(SVM)................................... 18
3.3.1 SVMIntuition ........................................ 18
3.3.2 SVMinPython........................................ 18
3.3.3 SVMinR........................................... 18
3.4 KernelSVM.............................................. 19
Page 1 of 51