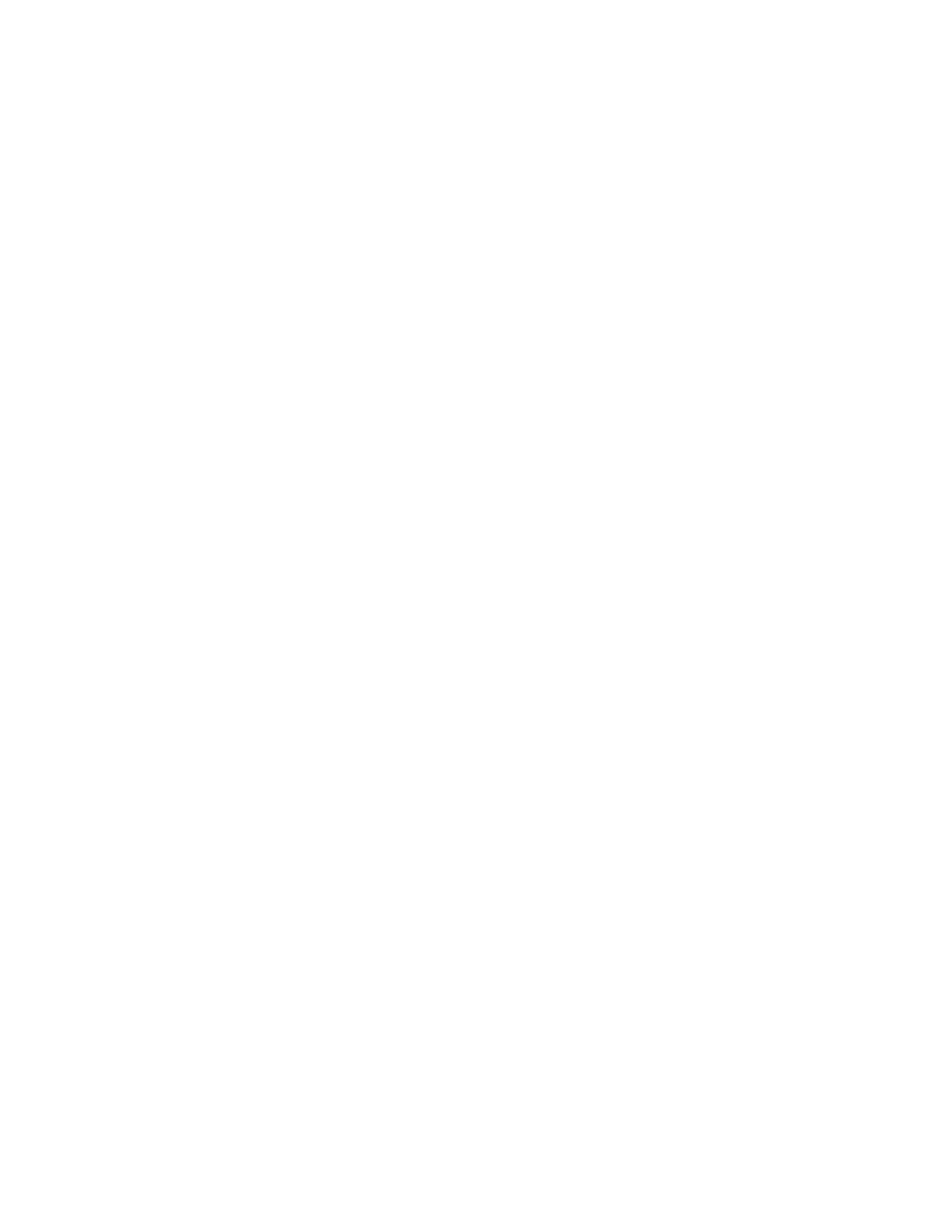
LCDWrLn2 ...................................................................................................................................24
LCDClear .....................................................................................................................................24
LCDClrLn1 ...................................................................................................................................24
LCDClrLn2 ...................................................................................................................................24
LCDWriteByte ..............................................................................................................................25
Bin2ASCII ....................................................................................................................................25
Data Manipulation............................................................................................................................26
Writing Your Name ..........................................................................................................................28
Write Up...........................................................................................................................................28
Additional Questions........................................................................................................................28
Challenge ........................................................................................................................................29
Lab 4 ...................................................................................................................................................30
Large Number Arithmetic ....................................................................................................................30
Objectives........................................................................................................................................31
Prelab ..............................................................................................................................................31
Procedure ........................................................................................................................................31
Assignment......................................................................................................................................33
Write Up...........................................................................................................................................34
Additional Questions........................................................................................................................34
Challenge ........................................................................................................................................34
Lab 5 ...................................................................................................................................................37
Simple Interrupts .................................................................................................................................37
Objectives........................................................................................................................................38
Prelab ..............................................................................................................................................38
Introduction......................................................................................................................................39
Interrupting a TekBot .......................................................................................................................39
Write Up...........................................................................................................................................40
Additional Questions........................................................................................................................40
Challenge ........................................................................................................................................41
Lab 6 ...................................................................................................................................................43
Extremely Simple Computer (ESC).....................................................................................................43
Objectives........................................................................................................................................44
Comments .......................................................................................................................................44
Prelab ..............................................................................................................................................44
Specifications ..................................................................................................................................44
Data .............................................................................................................................................45
Registers......................................................................................................................................45
Memory ........................................................................................................................................46
Data Memory............................................................................................................................47