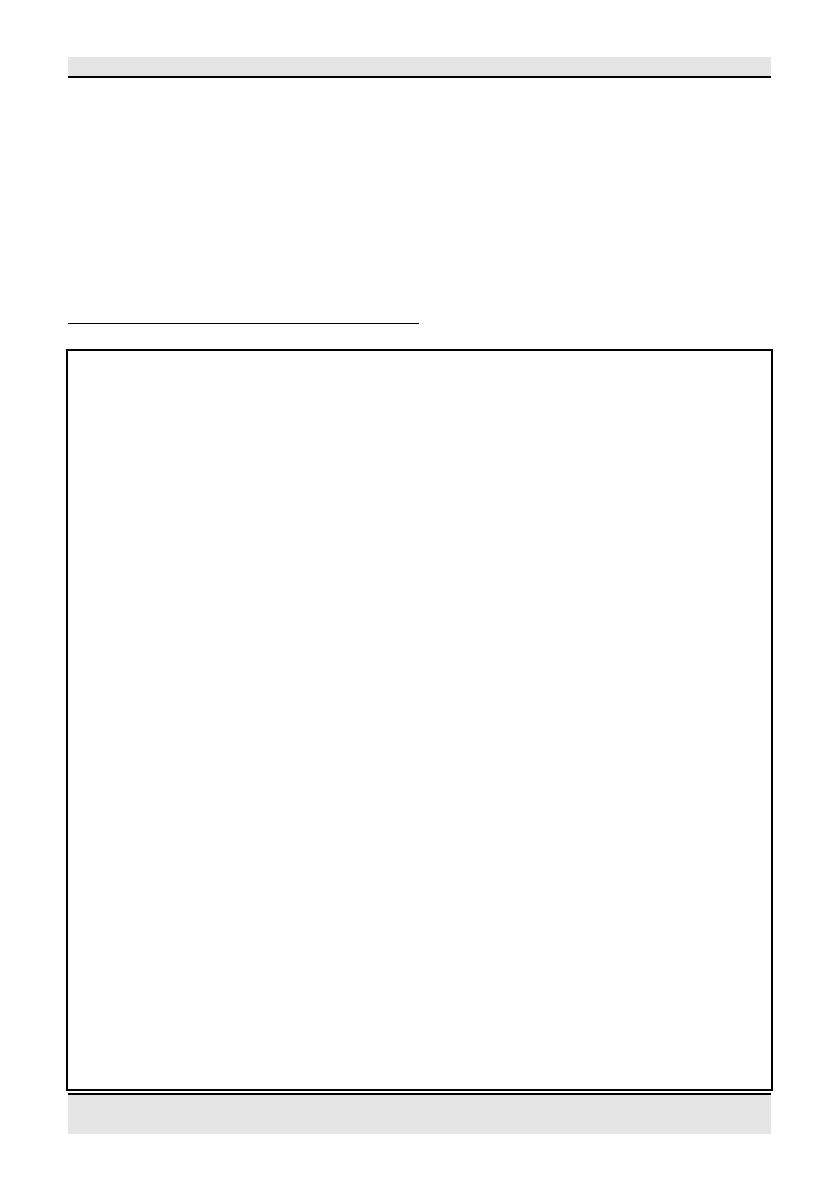
J.D.B. DIASOLUKA Nz.
Luyalu
JavaScript Tome-XXII
Functions
& Accessibilité éléments - 2/14 - dimanche, 14. octobre 2018 (3:41
pm)
deuxième paramètre [par défaut la base correspondant au format d’écri-
ture du nombre], l’entier qui commence une chaîne de caractères, et
l’affiche TOUJOURS en décimal.
parseFloat() : La fonction « parseFloat() » extrait le flottant qui
commence une chaîne de caractères.
toString() : La méthode « toString() » convertit le nombre en string et le
renvoie dans la base que vous spécifiez en paramètre.
Exemple, avec ou sans « use strict » :
<script> "use strict";
var pI=parseInt("0x10 heures 30 secondes")
// Lit 0x10 [en base utilisée {hexadécimale} (10h)]
// et l'affiche TOUJOURS en décimale (16d).
const h=parseInt("0100 jours",16)
// Lit 0100 en base Hexadécimale spécifiée
// et l'affiche TOUJOURS en décimale (16d).
let o=pI.toString(8)
// Lit 16d = 0x10 [TOUJOURS en décimale]
// et l'affiche en octale (020), comme spécifié.
const o1=parseInt("010")
// La base octale n'est pas reconnue en entrée.
// Lue en décimale.
const o2=parseInt("010",8)
// Lu en octale.
console.dir(pI+" | "+h+" | "+o+" | "+o1+" | "+o2)
var pF1=parseFloat("0x10 heures 30 secondes")
// Ne lit les hexédécimaux qu'avant le 'x'
// et affiche donc TOUJOURS zéro.
var pF2=parseFloat("16.85 heures",16)
// Lit 16.85 [TOUJOURS en décimal]
// et le renvoie TOUJOURS en décimale (16.50).
var pF3=pF2*2
// pF2 avait reçu en décimal.
let fo=pF2.toString(8)
// Lit 16.85d [TOUJOURS en décimale]
// et l'affiche en octale (20.663...), comme spécifié.
const fo1=parseFloat("010")
// La base octale n'est pas reconnue en entrée.
// Lue en décimale.