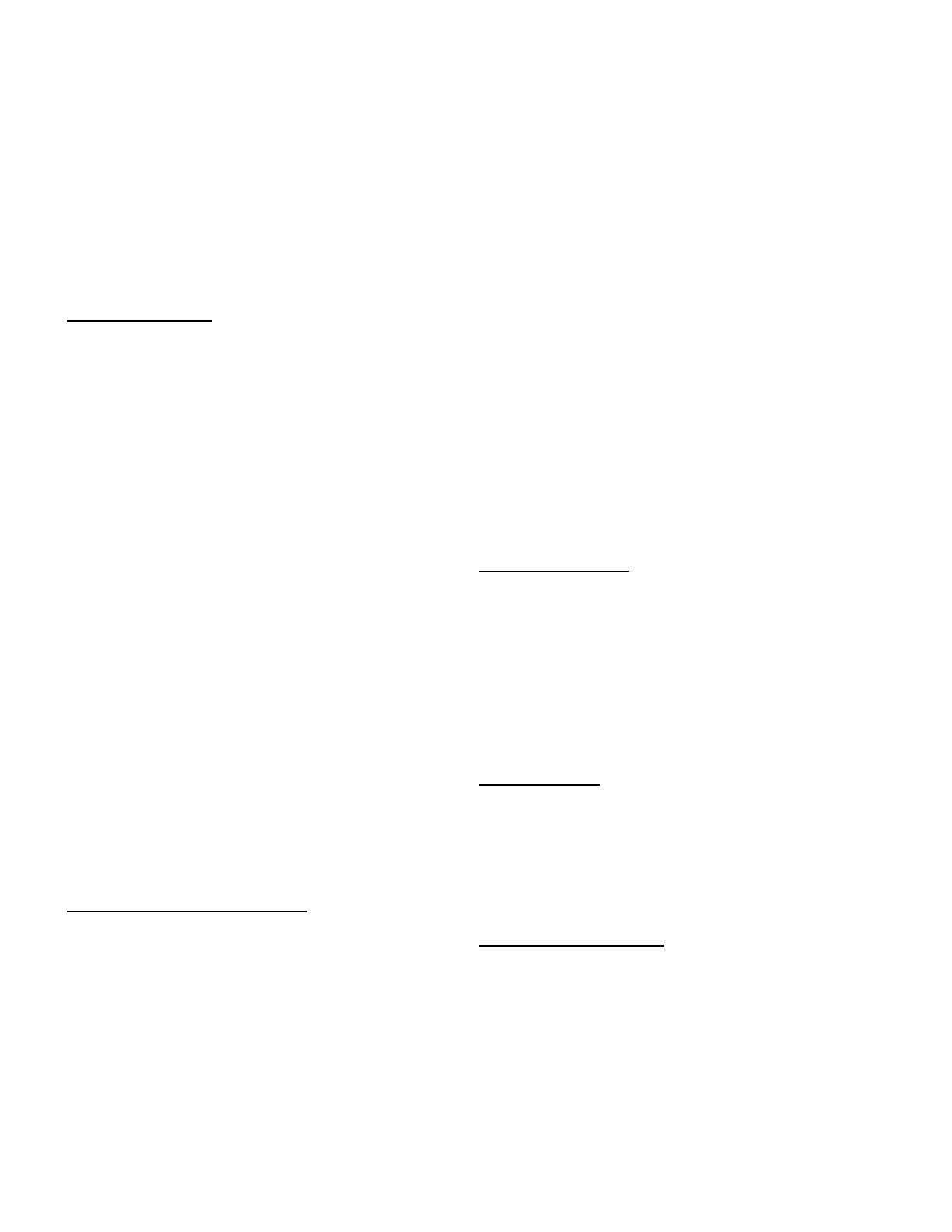
structures. The Tree methods include createNode, deleteNode,
setRoot, setLeave, create1stBranch, createBranch, and cre-
ateTree; the Graph methods include createUgraph, addEdge,
and getShortestPath; and the Binary Tree methods include
search, insert, delete and getRoot. It should be noted that the
linear and non-linear data structure components are separated
into 2 atomic domains for illustration purposes. If such con-
cepts are fully implemented in Java, many of the cumbersome
design and coding of applications can be eliminated. From a
programmer’s perspective, this means less coding effort and
fewer visible components to be accounted for.
GUI Atomic Domain: The GUI atomic domain and its wrap-
per, called GUIWrapper, include a number of GUI compo-
nents such as button, checkboxes, dropdown lists, menu, and
others. A GUI component, such as a button, can be associated
with several classes. To build a button, the developer has to
use a class to specify the color of the button, another class to
apply a border to the button, and another class to specify the
font style of the label or a class to specify an image picture for
the button, and so on. This lengthy process can be repeated to
build all the components that make up the application inter-
face. With atomic domains, wrapping related components into
an atomic domain can speed up the coding process signifi-
cantly. With the GUI components (classes) being wrapped
into one atomic domain, the application developer can use one
reference to interact with the atomic domain (GUIWrapper) to
build needed GUI components.
GUI components share common methods. For example, most
GUI components define methods to change background and
foreground colors, methods to modify label names, and oth-
ers. The GUIWrapper (implemented as a class) incorporates
most of these methods. This makes learning how to reuse GUI
components easier by giving the user an abstract view of the
GUIWrapper class with its methods that can provide the same
functionalities of all the methods of many different GUI com-
ponents. To motivate the wrapping of existing components
and retaining their original functionalities, the GUIWrapper
class defines methods such as: setBackgroundColor, setFor-
groundColor, setFont, setLabelName, setVisibility, isVisible,
setEnabled, isEnable, setNewSize, getText, addActionLis-
tener, and addFocusListener. These methods provide the same
functionalities as the individual methods.
Input/Output (IO) Atomic Domain: The IO atomic domain
and its wrapper, called IOWrapper and is implemented as a
class, includes a number of I/O classes such as File, ObjectIn-
putStream, ObjectOutputStream, FileInputStream, FileOut-
putStream, BufferedReader, BufferedWriter, FileReader,
FileWriter, DataInputStream, and DataOutputStream. Because
of the nature of Java and in order to build an application with
IO capabilities, the programmer has to use several library
classes, such as the File class, to associate a file name and
location with a File object during runtime. Also, InputStream
and OutputStream classes are used to allow the application to
read and write to a secondary storage device, and several
more classes are used to handle the buffering process. With
the IOWrapper and instead of having to decide how many
classes and which one to use for IO process, the application
developer can use one reference to the IOWrapper class and the
wrapper manager creates necessary I/O components for the ap-
plication. The listed IO components share common methods. For
example, these IO components provide a link between the run-
ning program and a file object on an IO device. These IO com-
ponents also have similar methods used to transfer data
(read/write) to and from a file located on the IO device. IO
classes do not have as many methods as the GUI classes because
they provide a different type of service. To demonstrate the
wrapping of existing components and retaining their original
functionalities, the IOWrapper class defines methods such as
readLine, read, writeFile, newLine, readFile, closeReader-
Stream, and closeWriterStream.
4 Illustrative Applications
Selected applications are described in this section to illus-
trate how Java atomic domains and their wrappers facilitate
effective reuse of components in the development of Java appli-
cations. The approach is to contrast programming effort (lines of
code) with and without using atomic domains. The selected ap-
plications are Nine Tail, Tree, Payroll, and Bank Account. These
applications use the Java atomic domains described above.
Nine Tail Application: The Nine Tail application uses Breadth-
First Search (BFS) algorithm to solve the Nail Tail problem.
Nine coins are placed in a three by three matrix with some fac-
ing up and others facing down. A legal move is to take any coin
that is facing up and reverse it, together with the coins adjacent
to it. The task is to find the minimum number of moves that lead
to all coins facing down. The problem is solved when all coins
are facing down. This application utilizes the NDSWrapper
class. Figures 1 and 2 show the source code before and after
using the NDSWrapper class in the solution. The coding effort in
Figure-2 is simpler than that in Figure-1.
Tree Application: The Tree application creates a folder struc-
ture and allows user to add, delete, and update values under a
specified node. This application utilizes the NDSWrapper class.
Figures 3 and 4 show the source code before and after using the
NDS atomic domain. In Figure-3 the user needs to explicitly
identify all the non-linear data structure components and their
types while in Figure-4 the user creates all the NDS objects from
the same wrapper class.
Bank Account Application: The Bank Account example illus-
trates the process of creating account objects and allowing the
user to save account information into a file. This application
utilizes the GUIWrapper, IOWrapper, and DSWrapper classes.
Figures 5 and 6 show partial code before and after using the
GUI, IO, and DS atomic domains. The omitted code in both
Figures is identical. In Figure-5, the user needs to explicitly
identify all required GUI, IO, and DS components and their
types; while in Figure-6 the user creates all GUI, IO, and DS
objects from respective same wrapper class. The coding effort in
Figure-6 is simpler than that in Figure-5.