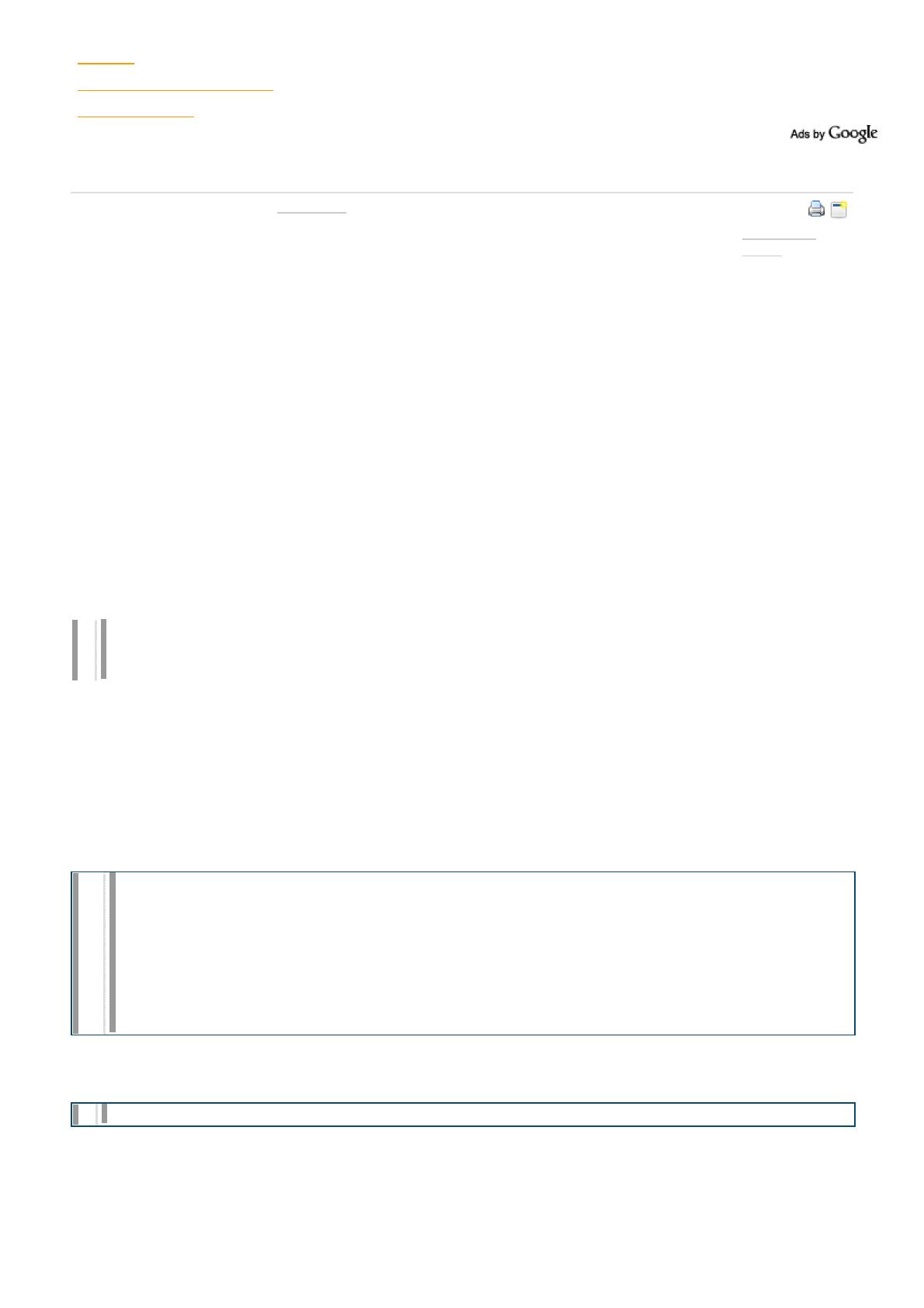
Monday, 19 July 2010 21:14 Garret Staus
[Report This
Article]
How to Create a Graph in Python
(0 votes, average 0 out of 5)
Python is an increasingly versatile language, and is frequently used for quickly writing up computation based programs. It can be
useful to graph input, and thankfully Python has one of the most convenient and accessible libraries to graph objects - Matplotlib.
Matplotlib is a plotting library for Python which extends the numpy library. Using matplotlib we can quickly create a graph of any
two lists. It is a very powerful library capable of making many types of graphs, including (but not limited to) bar graphs, scatter
plots, log graphs, and histograms. This tutorial will teach you how to very quickly make a graph in matplotlib. Note that matplotlib
is a very powerful library and this is just a scratch of the surface!
1. First, you will need to install matplotlib if you do not already have it. The matplotlib installation page provides a pretty
comprehensive and easy walkthrough on installing matplotlib (and numpy if you do not already have it).
http://matplotlib.sourceforge.net/users/installing.html
2. Now we're ready to write some code. Begin by setting up a new figure in matplotlib, making sure to import the library. We'll
also set up the "backend" of Matplotlib so we will be able to save the figure to our desired output.
1
2
3
import matplotlib.pyplot as plt
plt.figure()
3. Calling the figure() method sets up a new figure for our current plot. Depending on what kind of graph we want, we will call
a different method. Almost all graph types are called with two parameters for the x series and the y series, which are
simply lists in Python. Since most graphs are simple scatter plots, we will look at an example using plot(). In the example
below, we declare each series statically. Typically, one list will be some computation of the x_series. To add multiple lines
to a graph, simply call plot() again. If you want to create a new graph, call figure() again.
4
5
6
7
8
9
10
11
#create some data
x_series = [0,1,2,3,4,5]
y_series_1 = [x**2 for x in x_series]
y_series_2 = [x**3 for x in x_series]
#plot the two lines
plt.plot(x_series, y_series_1)
plt.plot(x_series, y_series_2)
4. Now we have plotted some data to our figure. But how do we see it? The most practical way is to save it to a .PDF or .PNG.
8plt.savefig("example.png")
5. Open the file you just saved to see your graph!
Logilab Spécialiste de Python et du Libre pour l'informatique scientifique www.logilab.fr
Talend: ETL Open Source Téléchargement 100% gratuit : ETL open source toute source de données
download.Talend.com/Open-Studio
Ellexus Breeze Advanced Script Debugging Linux build tracing for any flow! www.ellexus.com
14/02/2011 How to Create a Graph in Python - The…
thetechrepo.com/…/465-how-to-creat… 1/4