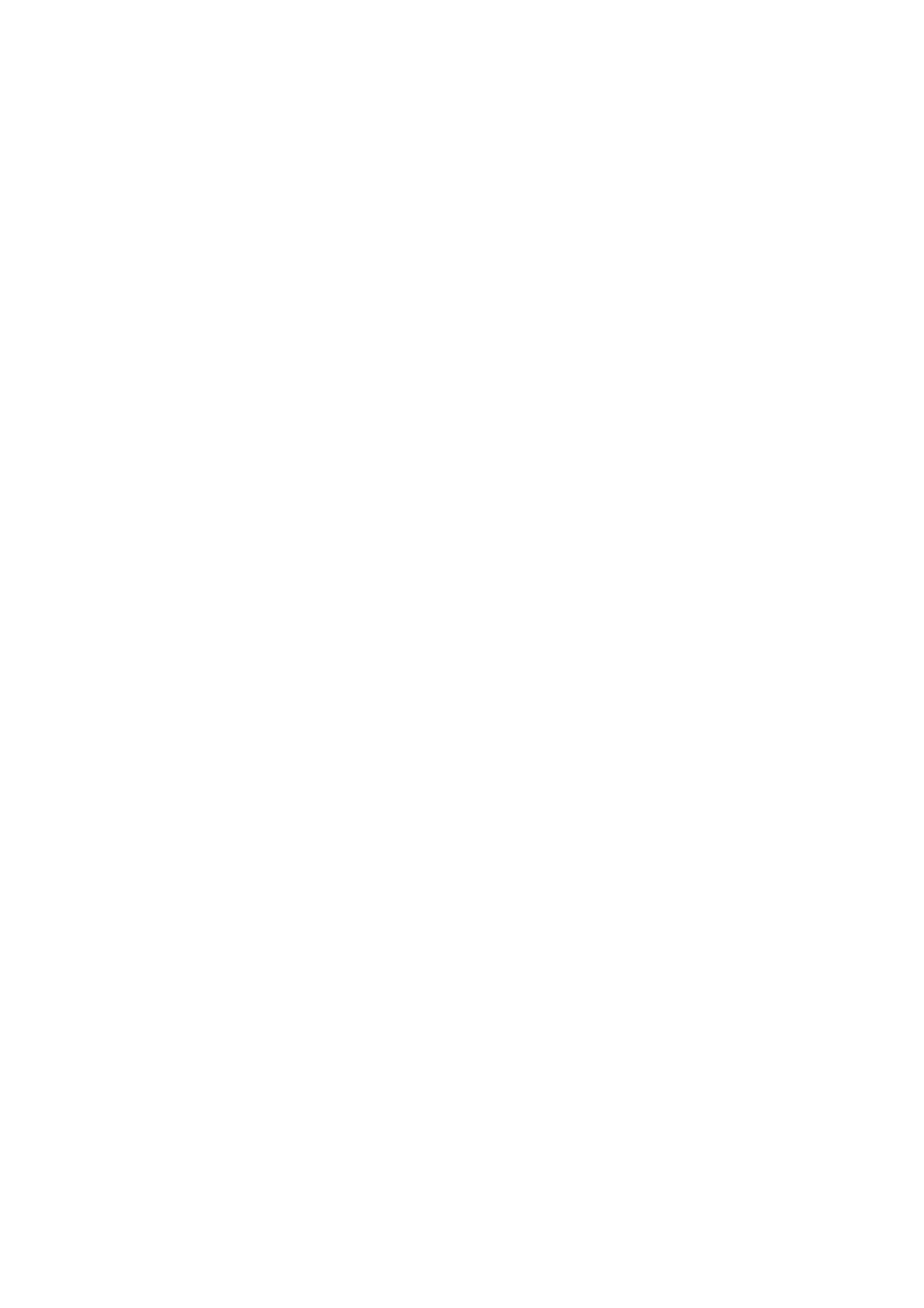
double argum = yy/Math.cos(phi);
double theta = Math.asin(argum); // If xx==0.0d, then theta is
NaN !
String nivString;
Graphics2D g2D = (Graphics2D)g.create(); // le
<code>Graphics<code> pour dessiner les échelons d'attitude
g2D.setColor(Color.WHITE);
g2D.scale(scaleX,scaleY);
g2D.translate(-bugXmin, -bugYmin);
g2D.rotate(theta);
for (int j = 0; j<10 ; j++) {
int niveau = 10*j;
double decal = 20*j;
nivString = '+'+Integer.toString(niveau);
g2D.translate(0,-floatScale*decal);
g2D.drawLine(-100, 0, 100, 0);
g2D.drawString(nivString,
-100-5-this.bugFM.stringWidth(nivString), 0);
g2D.drawString(nivString, 100+5, 0);
g2D.translate(0,2*floatScale*decal);
g2D.drawLine(-100, 0, 100, 0);
nivString = '-'+Integer.toString(niveau);
g2D.drawString(nivString,
-100-5-this.bugFM.stringWidth(nivString), 0);
g2D.drawString(nivString, 100+5, 0);
g2D.translate(0,-floatScale*decal);
}
}
}
/**
* Creates a panel where crosses will be drawn
*/
public Bug221242Test() {
this.bugPane = new BugPanel();
}
/**
* Create the GUI and show it. For thread safety,
* this method should be invoked from the
* event-dispatching thread.
*/
private static void createAndShowGUI() {
//Create and set up the window.
JFrame frame = new JFrame("Bug 221242 test");
frame.setLocation(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
//Create and set up the content pane.
Bug221242Test bugTest = new Bug221242Test();