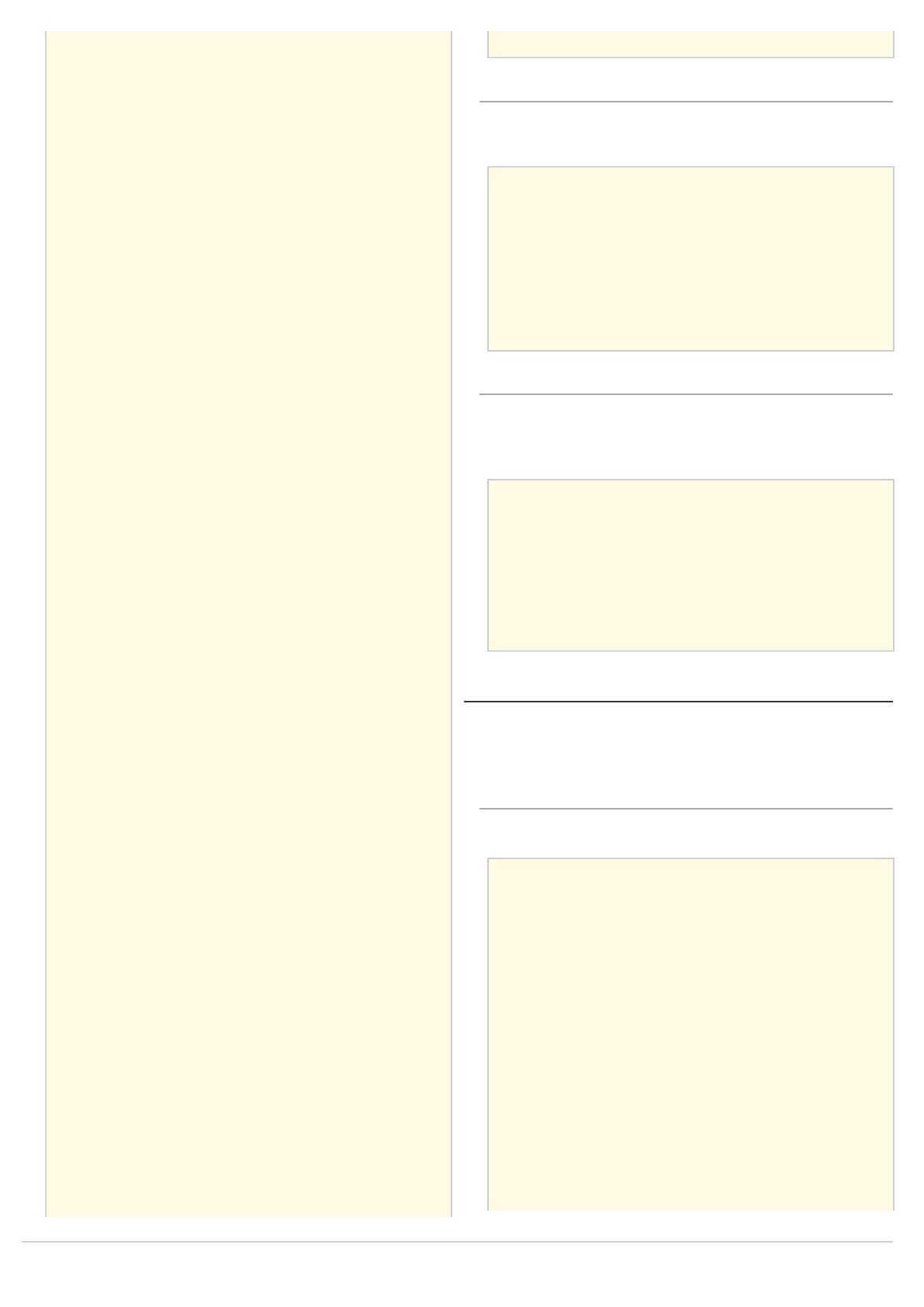
Utiliser plusieurs bases de données avec Spring/JPA/Hibernate
http://thegeekcorner.free.fr 2
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-
beans-3.0.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-
tx-3.0.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-
aop-3.0.xsd">
<bean class="org.springframework.orm.jpa.support.
PersistenceAnnotationBeanPostProcessor" />
<tx:annotation-driven />
<bean id="entityManagerFactory"
class="org.springframework.orm.jpa.\
LocalContainerEntityManagerFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="persistenceUnitName" value="jpaPU" />
<property name="jpaVendorAdapter">
<bean class="org.springframework.orm.jpa.vendor.\
HibernateJpaVendorAdapter">
<property name="database" value="POSTGRESQL" />
<property name="databasePlatform"
value="org.hibernate.dialect.PostgreSQLDialect" />
</bean>
</property>
</bean>
<bean id="entityManagerFactoryBis"
class="org.springframework.orm.jpa.\
LocalContainerEntityManagerFactoryBean">
<property name="dataSource" ref="dataSourceBis" />
<property name="persistenceUnitName" value="jpaPUBis" /
>
<property name="jpaVendorAdapter">
<bean class="org.springframework.orm.jpa.vendor.\
HibernateJpaVendorAdapter">
<property name="database" value="POSTGRESQL" />
<property name="databasePlatform"
value="org.hibernate.dialect.PostgreSQLDialect" />
</bean>
</property>
</bean>
<bean class="org.springframework.orm.jpa.support.\
PersistenceAnnotationBeanPostProcessor" />
<bean id="dataSource"
class="org.springframework.jdbc.datasource.\
DriverManagerDataSource">
<property name="driverClassName"
value="org.postgresql.Driver" />
<property name="url" value="jdbc:postgresql://
localhost:5432/schemaA" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
</bean>
<bean id="dataSourceBis"
class="org.springframework.jdbc.datasource.\
DriverManagerDataSource">
<property name="driverClassName"
value="org.postgresql.Driver" />
<property name="url" value="jdbc:postgresql://
localhost:5432/schemaB" />
<property name="username" value="${jdbc.username}" />
<property name="password" value="${jdbc.password}" />
</bean>
<bean id="hibernateDialect"
class="org.springframework.orm.jpa.vendor.HibernateJpaDialect" /
>
<bean id="transactionManager"
class="org.springframework.orm.jpa.JpaTransactionManager">
<property name="entityManagerFactory"
ref="entityManagerFactory" />
<property name="dataSource" ref="dataSource" />
<property name="jpaDialect" ref="hibernateDialect" />
<qualifier value="schemaA"/>
</bean>
<bean id="transactionManagerBis"
class="org.springframework.orm.jpa.JpaTransactionManager">
<property name="entityManagerFactory"
ref="entityManagerFactoryBis" />
<property name="dataSource" ref="dataSourceBis" />
<property name="jpaDialect" ref="hibernateDialect" />
<qualifier value="schemaB"/>
</bean>
</beans>
Business.xml
Ce fichier permet d’instancier les objets de votre
couche service
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-
beans-3.0.xsd
>
<bean id="puServiceA"
class="net.thegeekcorner.PUServiceA" />
<bean id="puServiceB"
class="net.thegeekcorner.PUServiceB” />
</beans>
applicationContext.xml
Dans ce fichier on inclus les 2 fichiers de
configurations XML liés à la couche service et le
mécanisme de persistence
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-
beans-3.0.xsd
">
<import resource="classpath:/META-INF/spring-
persistence.xml" />
<import resource="classpath:/META-INF/business.xml" />
</beans>
Les classes JAVA
Créer un nouveau projet avec comme package
principal net.thegeekcorner
Création des Entités persistentes
ClasseA.java
package net.thegeekcorner;
import java.util.LinkedList;
import java.util.List;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class ClassA {
private String attributAa;
private String attributAb;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getAttributAa(){