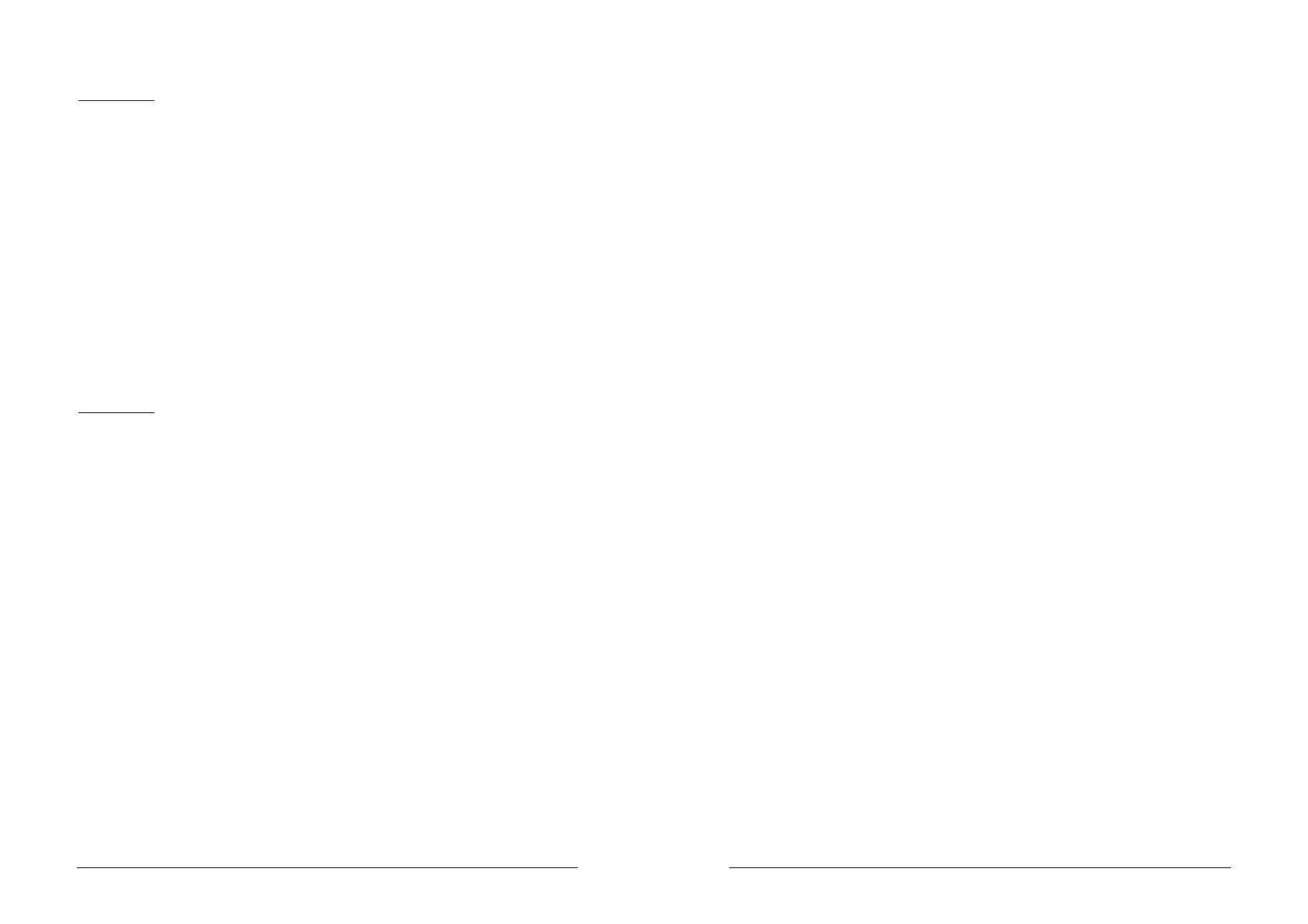
©Laura Perret 5 / 31 05.11.2006
Exercice 14
Code
int[] tableau = new int[10];
tableau[0] = 1;
tableau[1] = 1;
for (int i = 2; i < tableau.length; i++)
tableau[i] = tableau[i-2] + tableau[i-1];
System.out.println ("Suite de Fibonacci\n");
for (int i = 0; i < tableau.length; i++)
System.out.print (tableau[i] + " ");
System.out.println ();
Résultat
Suite de Fibonacci
1 1 2 3 5 8 13 21 34 55
Exercice 15
Code
int[][] matrice1 = new int[3][3];
int[][] matrice2 = new int[3][3];
int[][] matrice3 = new int[3][3];
int compteur = 0;
int somme = 0, produit = 0;
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
matrice1[i][j] = compteur++;
for (int i = 0; i < 3; i++)
for (int j = 0; j < 3; j++)
matrice2[i][j] = compteur--;
System.out.println ("Matrice 1\n");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++)
System.out.print(matrice1[i][j] + " ");
System.out.println ();
}
©Laura Perret 6 / 31 05.11.2006
System.out.println ("\nMatrice 2\n");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++)
System.out.print(matrice2[i][j] + " ");
System.out.println ();
}
for (int i = 0; i < 3; i++) { // ligne de m1
for (int j = 0; j < 3; j++) { // colonne de m2
for (int k = 0; k < 3; k++) { // element variable
produit = matrice1[i][k] * matrice2[k][j];
somme += produit;
}
matrice3[i][j] = somme;
somme = 0;
}
}
System.out.println ("\nMatrice 3\n");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++)
System.out.print(matrice3[i][j] + " ");
System.out.println ();
}
Résultat
Matrice 1
0 1 2
3 4 5
6 7 8
Matrice 2
9 8 7
6 5 4
3 2 1
Matrice 3
12 9 6
66 54 42
120 99 78