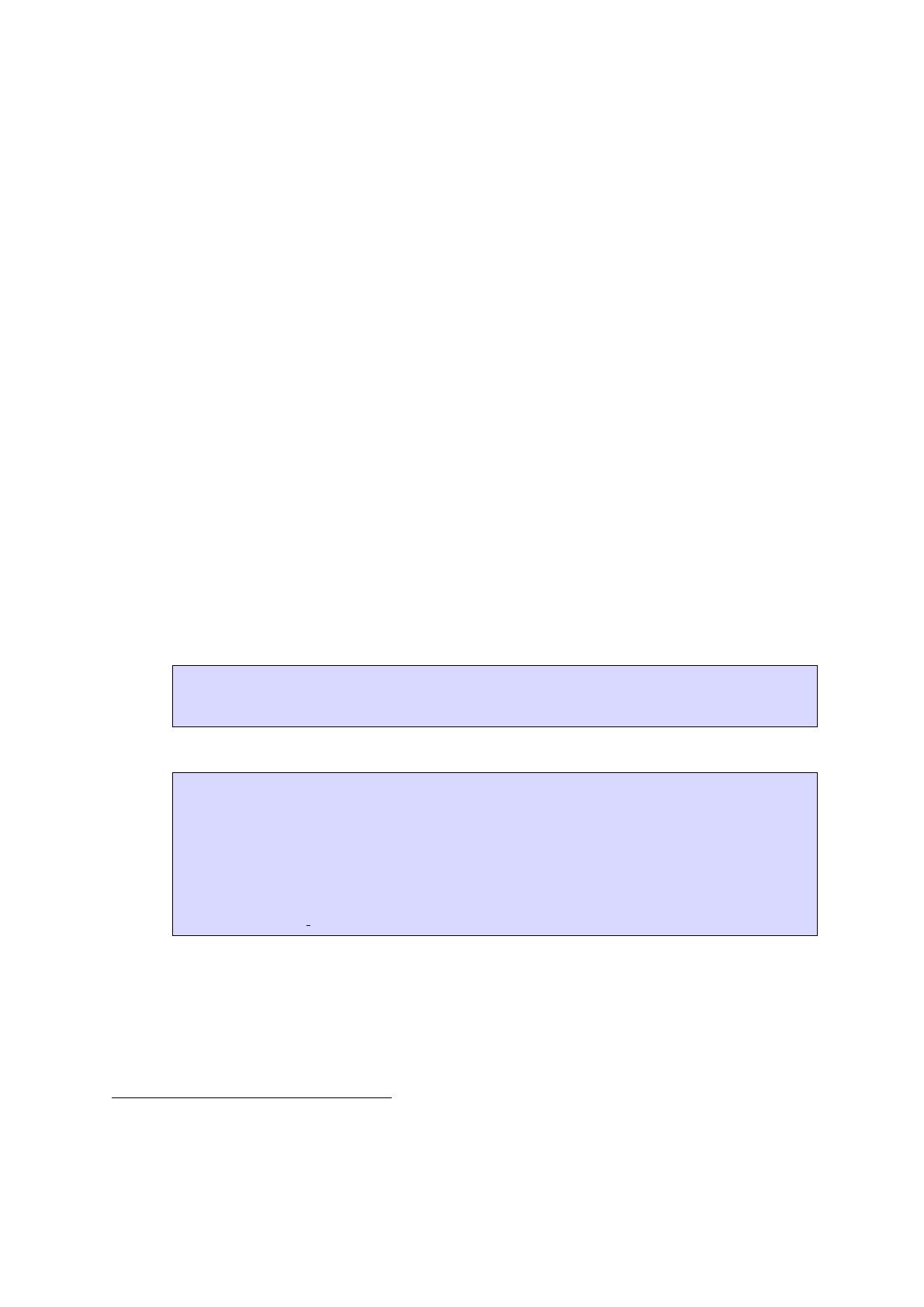
2 Some loops
Aims: write for-loops, learn to use range, write while-loops
Q1 In a script called “loops.py”, write 4 for loops1that
•print the numbers 0. . . 4 (without the range function)
•print the numbers 0. . . 4 (use the range function; see the CM for an example)
•print the numbers 4. . . 10 (again with range)
•print the numbers 4, 7, 10 ,13 ,16 (again with range)
Q2 Print the same sequence of numbers above, but now with while loops.
3 Count numbers in a list
Aims: use a for-loop in combination with an if statement (your first algorithm!)
Q3 Write, in natural language, an algorithm that counts the number of occurences of a number
in a list.
Q4 Program this algorithm in a Python script called occurencecount.py You can loop over the
items in a list as follows:
l = [ 1 , 8 , 3 , 2 , 7 , 2 , 7 , 2]
fo r i tem i n l :
# Do som ething w i t h ” i tem ” here .
occurencecount.py
# I n p u t
a=2 # Number t o search f o r
l = [ 1 , 8 , 3 , 2 , 7 , 2 , 7 , 2] # A l i s t o f numbers
# Al g o r i t hm
# Outpu t : number o f t im es ’ a ’ o ccurs i n ’ l ’
# For in st an ce , i n the l i s t above , ’ 2 ’ occurs 3 times
print ( occurence count )
1Hint: a typical error that people make is to forget the ‘:’ after the for loop definition, i.e. it is ‘for i in [0,1,2]:’
and not ‘for i in [0,1,2]’ The latter will make the Python interpreter raise an error ‘SyntaxError: invalid
syntax’.
2