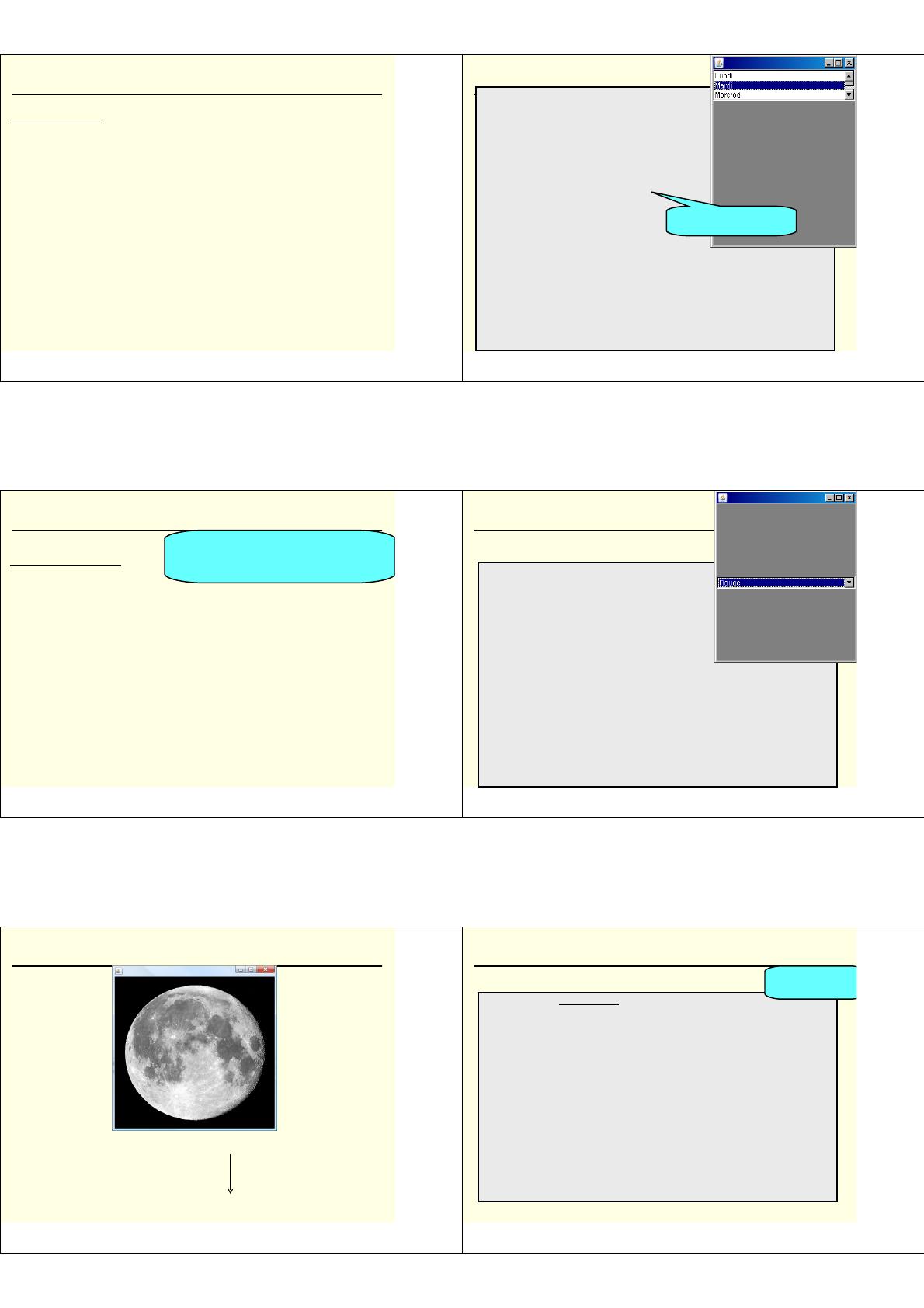
List
java.awt.List :
Liste déroulante. L'utilisateur peut choisir un ou plusieurs éléments.
Constructeurs :
• List( ), List(int rows), List(int rows, boolean multiple)
• Méthodes
• void addItem(String item), void addItem(String item, int n)
• void replaceItem(String item, int n), void delItem(int pos)
• int getItemCount(), String getItem(int n), String[] getItems()
• boolean isMultipleMode( )
• void setMultipleMode(boolean checked)
• void select (int pos), void deselect (int pos)
• String getSelectedItem( ), String[] getSelectedItems( )
• int getSelectedIndex( ), int[] getSelectedIndexes( )
• boolean isIndexSelected(int pos)
List
Exemple
import java.awt.*;
public class ListeDéroulante
extends Frame {
public ListeDéroulante(){
this.setSize(300, 250);
this.setBackground(Color.GRAY);
this.setLayout(new BorderLayout());
List lst = new List(3, false);
lst.add("Lundi");
lst.add("Mardi");
lst.add("Mercredi");
lst.add("Jeudi");
lst.add("Vendredi");
this.add(lst,"North");
this.setVisible(true);
}
public static void main(String[] args) {
new ListeDéroulante();
}
}
false, un seul item
peut être sélectionné
Choice
java.awt.Choice :
Répresente les pop-up menu.
• Constructeurs :
• Choice( )
• Méthodes de gestion du menu
• void addItem(String item), void insert(String item, int n)
• void remove(String item), void remove(int pos)
• int getItemCount(), String getItem(int n)
• Méthodes de gestion de la séléction
• void select (int pos), void select (String item)
• String getSelectedItem( )
• int getSelectedIndex( )
• boolean isIndexSelected(int pos)
List est similaire à Choice.
List permet éventuellement d'afficher et
sélectionner simultanément plusieurs items.
Choice
Exemple :
import java.awt.*;
public class ListeChoix extends Frame {
public ListeChoix(){
this.setSize(300, 250);
this.setBackground(Color.GRAY);
this.setLayout(new BorderLayout());
Choice choixCouleur = new Choice();
choixCouleur.add("Rouge");
choixCouleur.add("Bleu");
choixCouleur.add("Blanc");
this.add(choixCouleur, "Center");
this.setVisible(true);
}
public static void main(String[] args) {
new ListeChoix();
}
}
Exemple Insertion d'une image (1/3)
Résultat :
Hiérarchie de la composition: Frame ou Applet (BorderLayout)
Canvas
CENTER
jarExecutable
Exemple Insertion d'une image (2/3)
Code Frame :
public class ImageFrame extends Frame {
public ImageFrame() {
this.setSize(500,500);
this.setLayout(new BorderLayout());
Image lune = null;
try {
lune = ImageIO.read(
this.getClass().getResourceAsStream( "lune.jpg"));
} catch (IOException e) {
e.printStackTrace();
}
ImageCanvas ic = new ImageCanvas(lune);
add(ic,"Center");
this.setVisible(true);
this.addWindowListener(new WindowCloser(this));
}
C'est similaire
pour une Applet