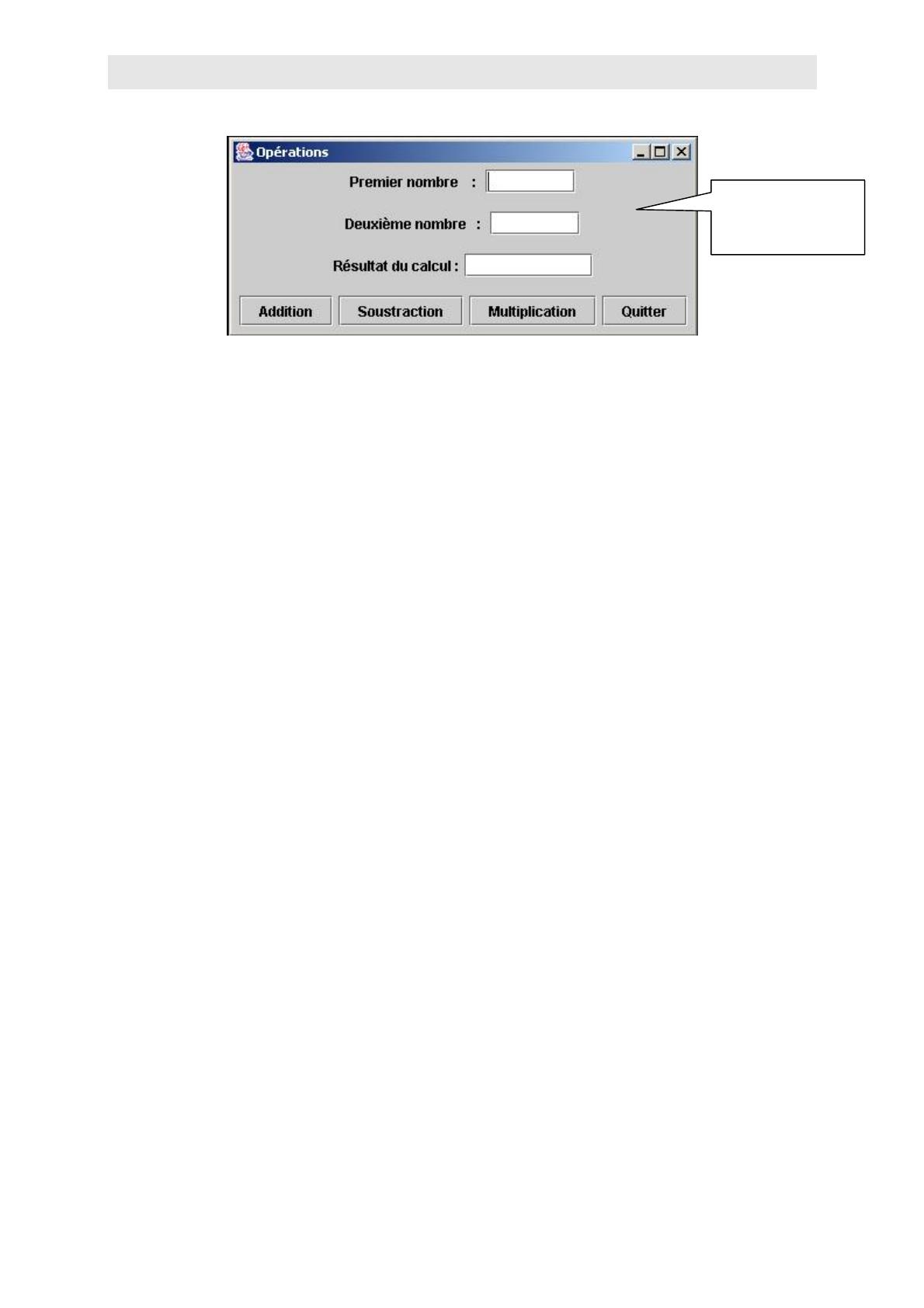
Les interfaces graphiques en JAVA
-1-
•Exemple 1 :
// --- swgop1.java
import java.io.*;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Fenetre5 extends JFrame implements ActionListener
{private JTextField champ_nb1, champ_nb2, champ_res;
private JButton b_plus, b_moins, b_mult, b_quitte;
public Fenetre5()
{setTitle("Opérations");
Container ccf = this.getContentPane();
ccf.setLayout(new GridLayout(4,1));
JPanel p1 = new JPanel();
p1.add(new JLabel("Premier nombre : "));
champ_nb1 = new JTextField(7);
p1.add(champ_nb1);
ccf.add(p1);
JPanel p2 = new JPanel();
p2.add(new JLabel("Deuxième nombre : "));
champ_nb2 = new JTextField(7);
p2.add(champ_nb2);
ccf.add(p2);
JPanel p3 = new JPanel();
p3.add(new JLabel("Résultat du calcul :"));
champ_res = new JTextField(10);
p3.add(champ_res);
ccf.add(p3);
JPanel p4 = new JPanel();
p4.setLayout(new FlowLayout(FlowLayout.RIGHT));
b_plus = new JButton("Addition");
b_moins = new JButton("Soustraction");
b_mult = new JButton("Multiplication");
b_quitte = new JButton("Quitter");
p4.add(b_plus); p4.add(b_moins);
p4.add(b_mult); p4.add(b_quitte);
ccf.add(p4);
b_plus.addActionListener(this);
b_moins.addActionListener(this);
b_mult.addActionListener(this);
b_quitte.addActionListener(this);
addWindowListener(new Delegue_Fen());
3 opérations sur
2 nombres