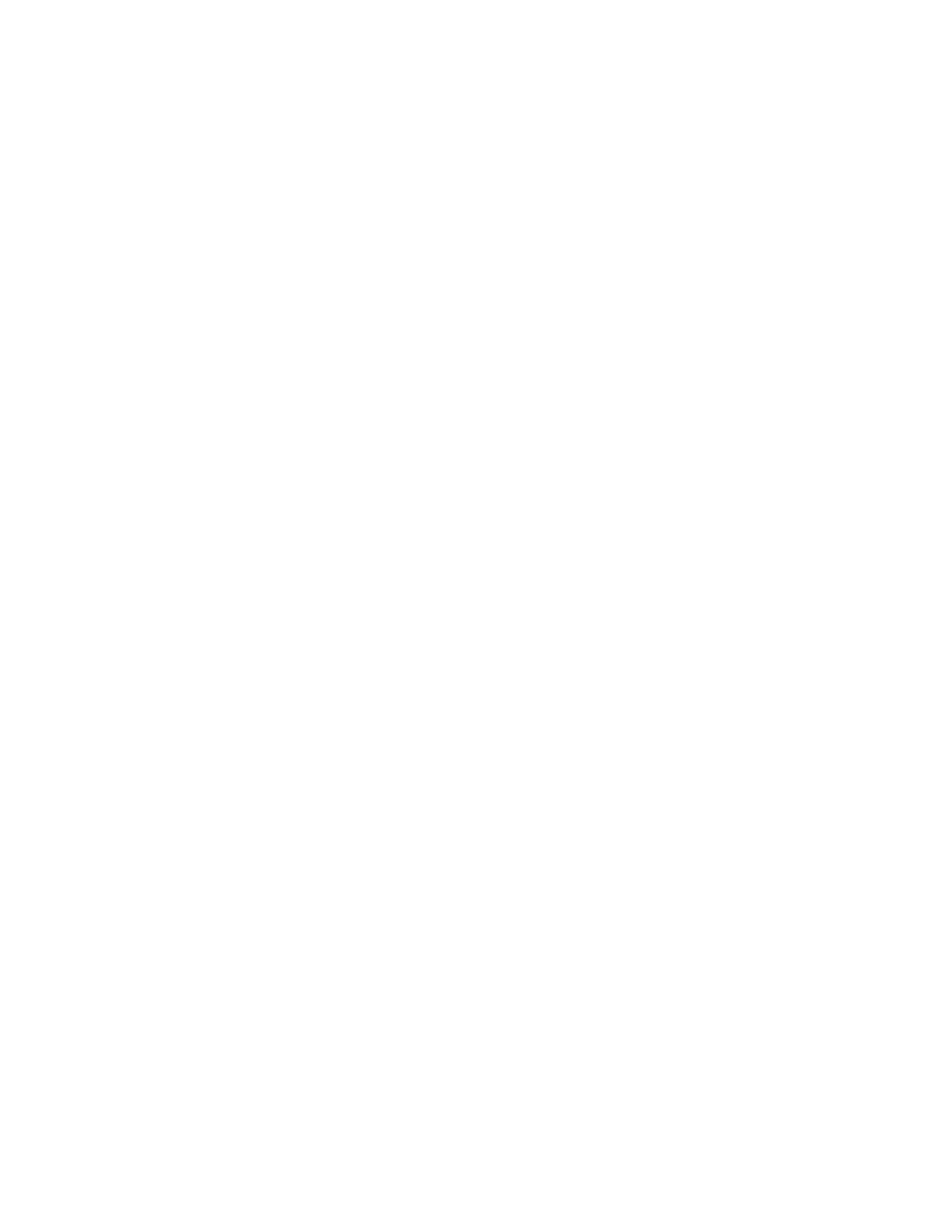
1.2 Calcul vectoris´e
In [8]: from math import cos
print cos(a)
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-8-aaa6d586df86> in <module>()
1 from math import cos
----> 2 print cos(a)
TypeError: only length-1 arrays can be converted to Python scalars
In [5]: from numpy import cos
c=cos(a)
print c[:10]
[ 0.97542417 0.9559976 0.889291 0.98941373 0.8121354 0.93883746
0.7051168 0.7920507 0.9395283 0.6542547 ]
In [10]: (a+a)[:10]
Out[10]: array([ 0.61851293, 1.73521928, 0.97038485, 1.54822963, 0.77315354,
1.01872002, 1.17717045, 0.12074412, 0.53769763, 0.49657872])
1.2.1 Comparaison
In [13]: b=list(a)
print b[:10]
[0.3092564639249985, 0.86760964130719376, 0.48519242363955573, 0.77411481507324209, 0.38657676754846271, 0.50936000958515759, 0.58858522711366779, 0.060372059305616954, 0.26884881489477819, 0.24828936212184904]
In [11]: %timeit a**2
The slowest run took 16.04 times longer than the fastest. This could mean that an intermediate result is being cached
1000000 loops, best of 3: 1.93 µs per loop
In [10]: %timeit [x**2 for x in b]
1000 loops, best of 3: 274 µs per loop
In [11]: %%timeit
s=0
for x in b:
s = s + x**2
1000 loops, best of 3: 320 µs per loop
2