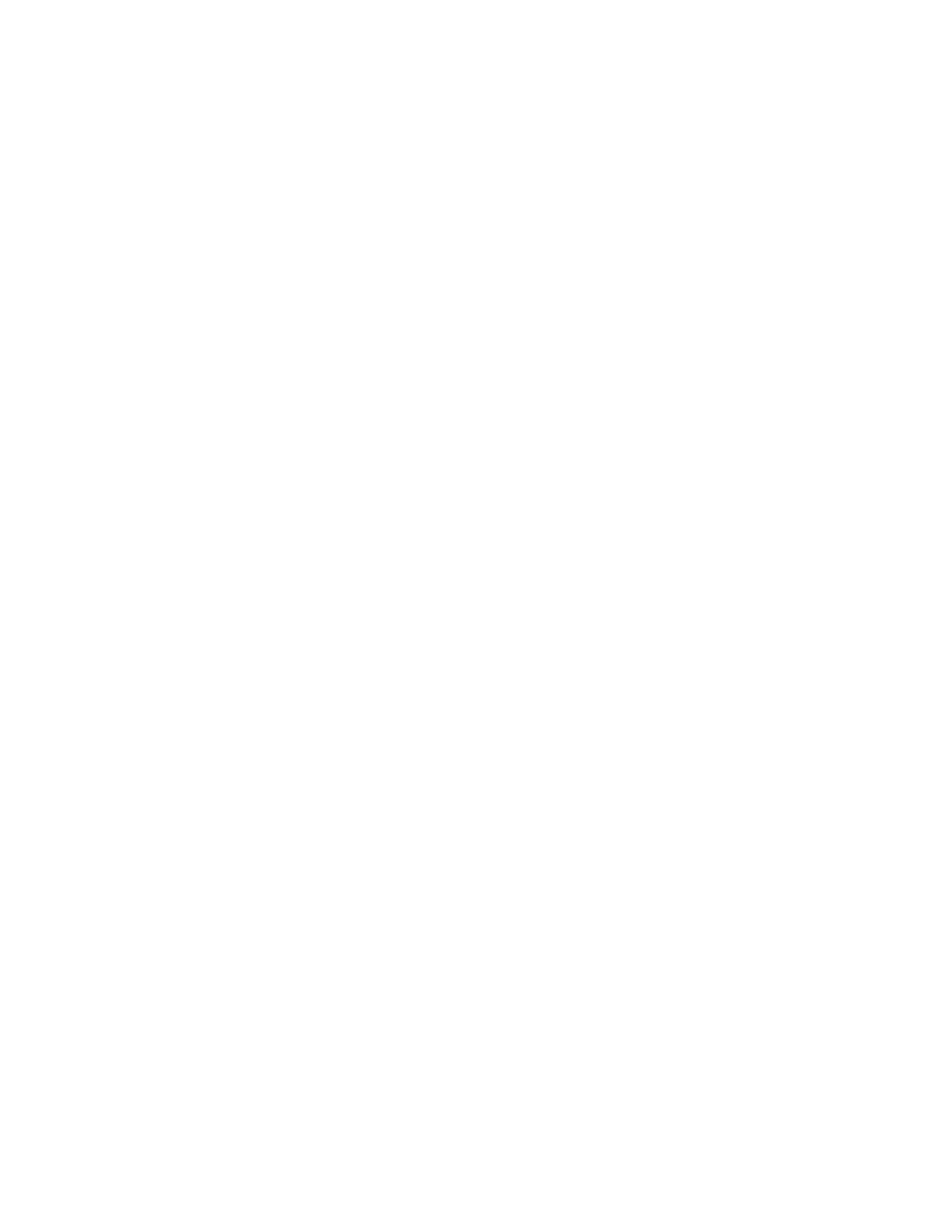
3
matA = affCase(matA, 1, 3, 1);
/**** modification de la troisieme ligne ******/
matA = affCase(matA, 2, 0, 1);
matA = affCase(matA, 2, 1, 2);
matA = affCase(matA, 2, 2, 1);
matA = affCase(matA, 2, 3, 0);
//affichage de la matrice matA apres creation et sans modification
printf("voici la matrice matA apres la modification : \n");
afficherMatrice(matA);
//recuperation d'un element de la matrice matA
caseA = recCase(matA, 2, 1);
printf("Voici la valeur de la case de matA choisie (L3/C2): %d\n", caseA);
//Creation de la matrice matB (3lignes/4colonnes) pour l'addition avec matA
printf("\n************ Matrice matB *****************\n");
matB=creerMatrice(3,4);
//modification de la matrice matB
/**** modification de la premiere ligne ******/
matB = affCase(matB, 0, 0, 1);
matB = affCase(matB, 0, 1, 0);
matB = affCase(matB, 0, 2, 0);
matB = affCase(matB, 0, 3, 2);
/**** modification de la deuxieme ligne ******/
matB = affCase(matB, 1, 0, 0);
matB = affCase(matB, 1, 1, 1);
matB = affCase(matB, 1, 2, 1);
matB = affCase(matB, 1, 3, 1);
/**** modification de la troisieme ligne ******/
matB = affCase(matB, 2, 0, 3);
matB = affCase(matB, 2, 1, 1);
matB = affCase(matB, 2, 2, 2);
matB = affCase(matB, 2, 3, 1);
//affichage de la matrice matB
printf("voici la matrice matB apres la modification : \n");
afficherMatrice(matB);
//addition de matA+matB
printf("\n************ matA + matB *****************\n");
matAdd = additionMatrice(matA, matB);
//affichage du resultat de l'addition
printf("l'addition entre matA et matB donne le resultat suivant : \n");
afficherMatrice(matAdd);
//Creation de la matrice matC (4lignes/2colonnes) pour la multiplication avec matA
printf("\n************ Matrice matC *****************\n");
matC=creerMatrice(4,2);
//modification de la matrice matB
/**** modification de la premiere ligne ******/
matC = affCase(matC, 0, 0, 1);
matC = affCase(matC, 0, 1, 0);
/**** modification de la deuxieme ligne ******/
matC = affCase(matC, 1, 0, 1);
matC = affCase(matC, 1, 1, 1);
/**** modification de la troisieme ligne ******/
matC = affCase(matC, 2, 0, 0);
matC = affCase(matC, 2, 1, 0);
/**** modification de la quatrieme ligne ******/
matC = affCase(matC, 3, 0, 0);
matC = affCase(matC, 3, 1, 1);
//affichage de la matrice matC
printf("voici la matrice matC apres la modification : \n");
afficherMatrice(matC);
//multiplication de matA*matC
printf("\n************ matA x matC *****************\n");
matMulti = multiplicationMatrice(matA, matC);
//affichage du resultat de la multiplication
printf("la multiplication entre matA et matC donne le resultat suivant : \n");
afficherMatrice(matMulti);
return (0);
}