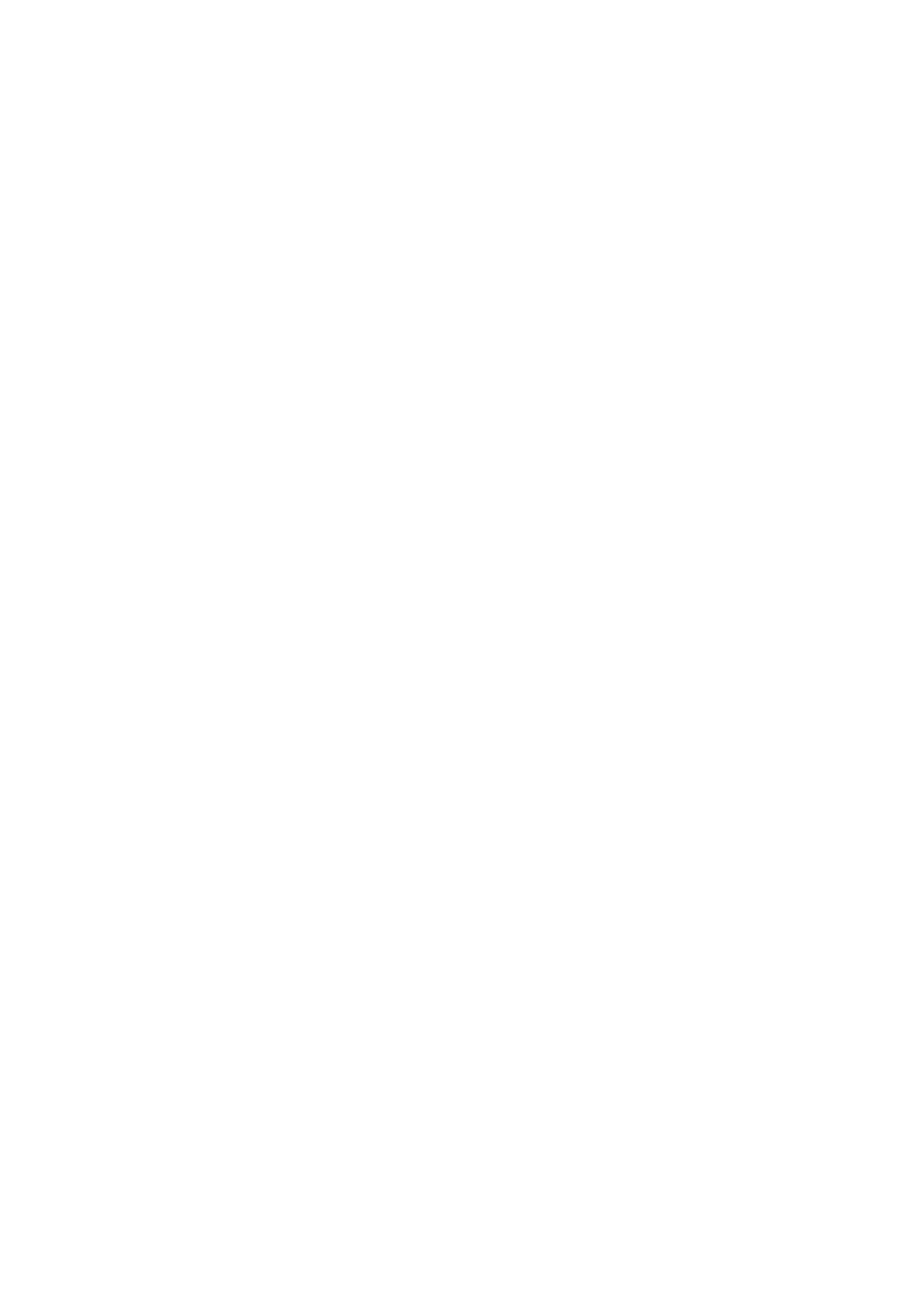
B lit a la fois les donnees en provenance de A et le clavier. Quand
il lit stop il ferme la socket
(e) Donner le code correspondant pour Aet B.
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class pairA2 {
public static void main (String[] args){
ServerSocket server;
int port=1027;
try {
// exception si le port est utilise
server = new ServerSocket(port);
Socket connection = server.accept( );
DataOutputStream outToClient =new DataOutputStream(connection.getOutputStream());
//lecture au clavier et envoie a pair B
String nom;
Scanner sc=new Scanner(System.in);
System.out.println("des lignes");
while(true)
{nom=sc.nextLine();
outToClient.writeBytes(nom+"\r\n");
}
} catch (IOException ex) {
System.err.println("sortie car la socket est ferme");
}
}
}
-----------
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.Socket;
import java.net.UnknownHostException;
public class pairB2 {
public static void main (String[] args){
BufferedReader networkIn = null;
try { // connexion avec le serveur
Socket connection = new Socket("localhost", 1027);
4