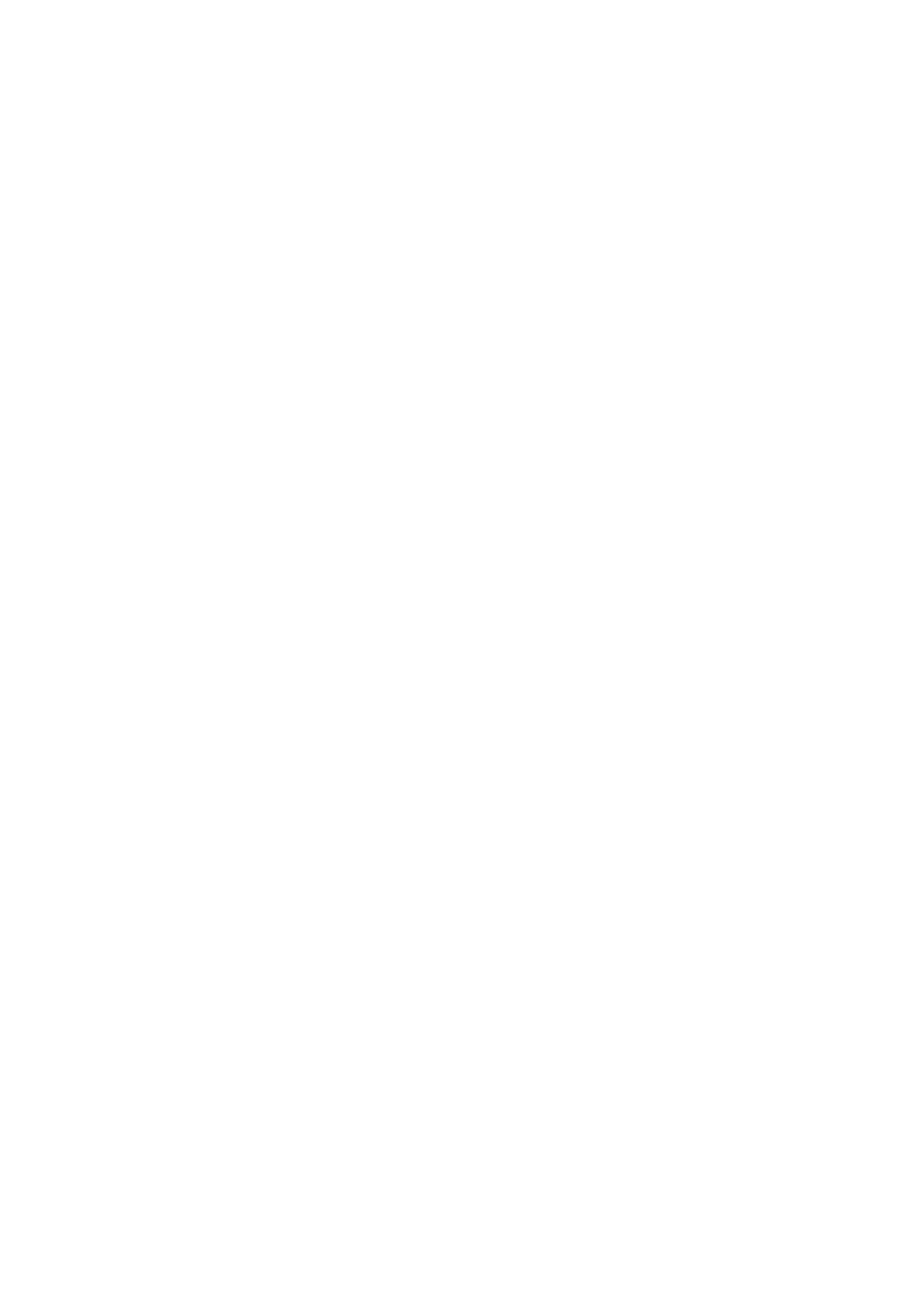
)&1?void 0:f&&(a[B]|0)&2?a:Ha(a,b,c,void 0!==d,e,f);else if(D(a)){const
h={};for(let l in a)h[l]=Ga(a[l],b,c,d,e,f);a=h}else a=b(a,d);return a}}
function Ha(a,b,c,d,e,f){const h=d||c?a[B]|0:0;d=d?!!(h&32):void
0;a=A(a);for(let
l=0;l<a.length;l++)a[l]=Ga(a[l],b,c,d,e,f);c&&c(h,a);return a}function
Ia(a){return a.G===ua?a.toJSON():Ea(a)};function
Ja(a,b,c=sa){if(null!=a){if(a instanceof Uint8Array)return b?a:new
Uint8Array(a);if(Array.isArray(a)){var d=a[B]|0;if(d&2)return
a;b&&(b=0===d||!!(d&32)&&!(d&64||!(d&16)));return b?(a[B]=(d|34)&-
12293,a):Ha(a,Ja,d&4?sa:c,!0,!1,!0)}a.G===ua&&(c=a.l,d=c[B],a=d&2?a:Ka(a,
c,d,!0));return a}}function
Ka(a,b,c,d){a=a.constructor;Da=b=La(b,c,d);b=new a(b);Da=void 0;return
b}function La(a,b,c){const
d=c||b&2?sa:ra,e=!!(b&32);a=Fa(a,b,f=>Ja(f,e,d));a[B]=a[B]|32|(c?2:0);ret
urn a}
function Ma(a){const b=a.l,c=b[B];return
c&2?Ka(a,b,c,!1):a};Object.freeze({});Object.freeze({});function
H(a,b){a=a.l;return Na(a,a[B],b)}function Na(a,b,c,d){if(-1===c)return
null;if(c>=ta(b)){if(b&256)return a[a.length-1][c]}else{var
e=a.length;if(d&&b&256&&(d=a[e-1][c],null!=d))return d;b=c+(+!!(b&512)-
1);if(b<e)return a[b]}}function Oa(a,b,c,d){var
e=ta(b);if(1>=e||d){d=b;if(b&256)e=a[a.length-1];else{if(null==c)return
d;e=a[e+(+!!(b&512)-1)]={};d|=256}e[1]=c;d!==b&&(a[B]=d);return
d}a[1+(+!!(b&512)-1)]=c;b&256&&(a=a[a.length-1],1 in a&&delete
a[1]);return b}
function Pa(a){var b=a.l,c=b[B],d=Na(b,c,1,!1);const
e=Ba(d,Qa,c);e!==d&&null!=e&&Oa(b,c,e,!1);b=e;if(null==b)return
b;a=a.l;c=a[B];c&2||(d=Ma(b),d!==b&&(b=d,Oa(a,c,b,!1)));return b}
function Ra(a){a=a.l;var
b=a[B],c=!!(2&b),d=b,e=c?1:2,f=Sa;b=1===e;e=2===e;var
h=!!(2&d)&&e,l=d&2,g=Na(a,d,1);Array.isArray(g)||(g=F);var
m=g[B]|0;0===m&&d&32&&!l?(m|=33,g[B]=m):m&1||(m|=1,g[B]=m);l&&(d=!1,m&2||
(g[B]|=34,d=!!(4&m)),d&&Object.freeze(g));d=a[B];var
k=g[B]|0,p=!!(2&k);m=!!(4&k);l=!!(32&k);let r=p&&m||!!(2048&k);if(!m){var
v=g,q=d;const w=!!(2&k);w&&(q=C(q,2,!0));let
x=!w,E=!0,Q=0,I=0;for(;Q<v.length;Q++){const J=Ba(v[Q],f,q);if(J
instanceof f){if(!w){const R=!!((J.l[B]|0)&2);x&&(x=!R);
E&&(E=R)}v[I++]=J}}I<Q&&(v.length=I);k=C(k,4,!0);k=C(k,16,E);k=C(k,8,x);v
[B]=k;p&&!h&&(Object.freeze(g),r=!0)}f=k;h=!!(8&k)||b&&!g.length;if(!c&&!
h){r&&(g=A(g),r=!1,f=0,k=Ta(k,d),d=Oa(a,d,g));c=g;h=k;for(p=0;p<c.length;
p++)v=c[p],k=Ma(v),v!==k&&(c[p]=k);h=C(h,8,!0);k=h=C(h,16,!c.length)}r||(
b?k=C(k,!g.length||16&k&&(!m||l)?2:2048,!0):k=C(k,32,!1),k!==f&&(g[B]=k),
b&&(Object.freeze(g),r=!0));e&&r&&(g=A(g),k=Ta(k,d),g[B]=k,Oa(a,d,g));ret
urn g}
function Ta(a,b){a=C(a,2,!!(2&b));a=C(a,32,!!(32&b)&&!1);return
a=C(a,2048,!1)}function
K(a,b){a=H(a,b);return(null==a||"boolean"===typeof a?a:"number"===typeof
a?!!a:void 0)??!1}function L(a,b){return Aa(H(a,b))??0};var
M=class{constructor(a){a:{null==a&&(a=Da);Da=void 0;if(null==a){var
b=96;a=[]}else{if(!Array.isArray(a))throw Error();b=a[B]|0;if(b&64)break
a;b|=64;var c=a.length;if(c&&(--c,D(a[c]))){b|=256;c-=+!!(b&512)-
1;if(1024<=c)throw Error();b=b&-
16760833|(c&1023)<<14}}a[B]=b}this.l=a}toJSON(){var a=Ha(this.l,Ia,void
0,void 0,!1,!1);return
Ua(this,a,!0)}};M.prototype.G=ua;M.prototype.toString=function(){return
Ua(this,this.l,!1).toString()};
function Ua(a,b,c){const d=a.constructor.N;var
e=(c?a.l:b)[B],f=ta(e),h=!1;if(d&&xa){if(!c){b=A(b);var
l;if(b.length&&D(l=b[b.length-
1]))for(h=0;h<d.length;h++)if(d[h]>=f){Object.assign(b[b.length-
1]={},l);break}h=!0}f=b;c=!c;l=a.l[B];a=ta(l);l=+!!(l&512)-1;var