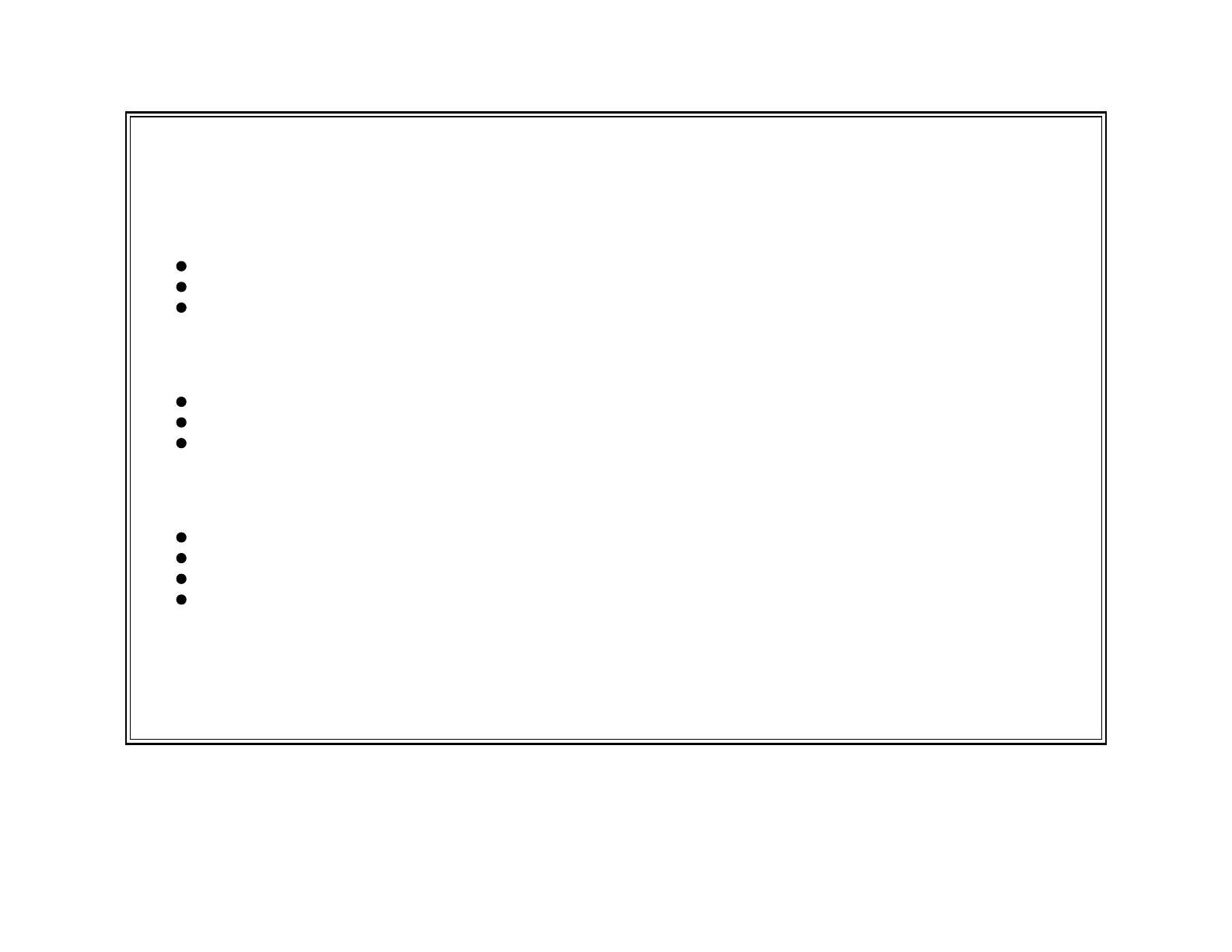
Preliminaries
Audience
Experienced programmers who are familiar with advanced programming topics in other languages.
Python programmers who want to know more.
Programmers who aren’t afraid of gory details.
Disclaimer
This tutorial is aimed at an advanced audience
I assume prior knowledge of topics in Operating Systems and Networks.
Prior experience with Python won’t hurt as well.
My Background
I was drawn to Python as a C programmer.
Primary interest is using Python as an interpreted interface to C programs.
Wrote the "Python Essential Reference" in 1999 (New Riders Publishing).
All of the material presented here can be found in that source.
O’Reilly OSCON 2000, Advanced Python Programming, Slide 3