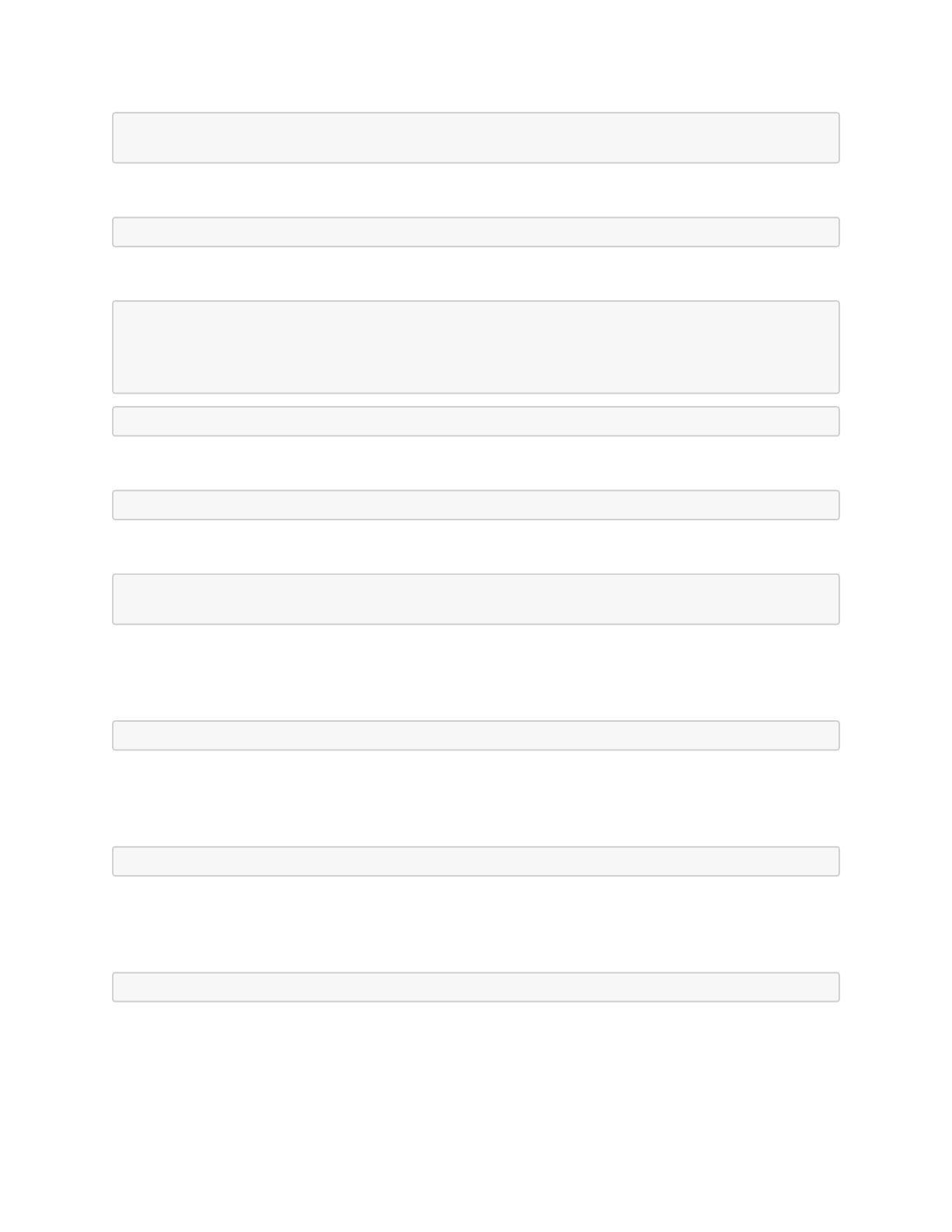
[31]: # In case we needed the Boolean Answer:
np.array_equal(A, B)
[31]: False
[32]: np.array_equal(A, A)
[32]: True
[36]: # Logical Operations: Or & And
# Lets Create Two arrays of Bool Values
b1 =np.array([0,1,0,1,1,1])
b2 =np.array([1,1,0,1,0,1])
[38]: np.logical_or(b1, b2)
[38]: array([ True, True, False, True, True, True])
[39]: np.logical_and(b1, b2)
[39]: array([False, True, False, True, False, True])
[40]: # Mapping Numpy Math-Built-in Functions all over elements
np.sin(A)
[40]: array([[ 0.90929743, -0.2794155 , -0.7568025 ],
[ 0.41211849, 0.84147098, 0.14112001],
[ 0.98935825, -0.95892427, 0.6569866 ]])
[41]: np.cos(B)
[41]: array([[ 0.96017029, -0.14550003, 0.54030231],
[ 0.75390225, -0.91113026, 0.28366219],
[-0.14550003, -0.41614684, -0.65364362]])
[42]: np.tan(A +B)
[42]: array([[ -6.79971146, 7.24460662, -3.38051501],
[ 0.30063224, 0.64836083, -6.79971146],
[ 0.30063224, 0.87144798, -225.95084645]])
[56]: np.sqrt(A +B)
[56]: array([[2.82842712, 3.74165739, 2.23606798],
[4. , 3.16227766, 2.82842712],
[4. , 2.64575131, 3.31662479]])
4