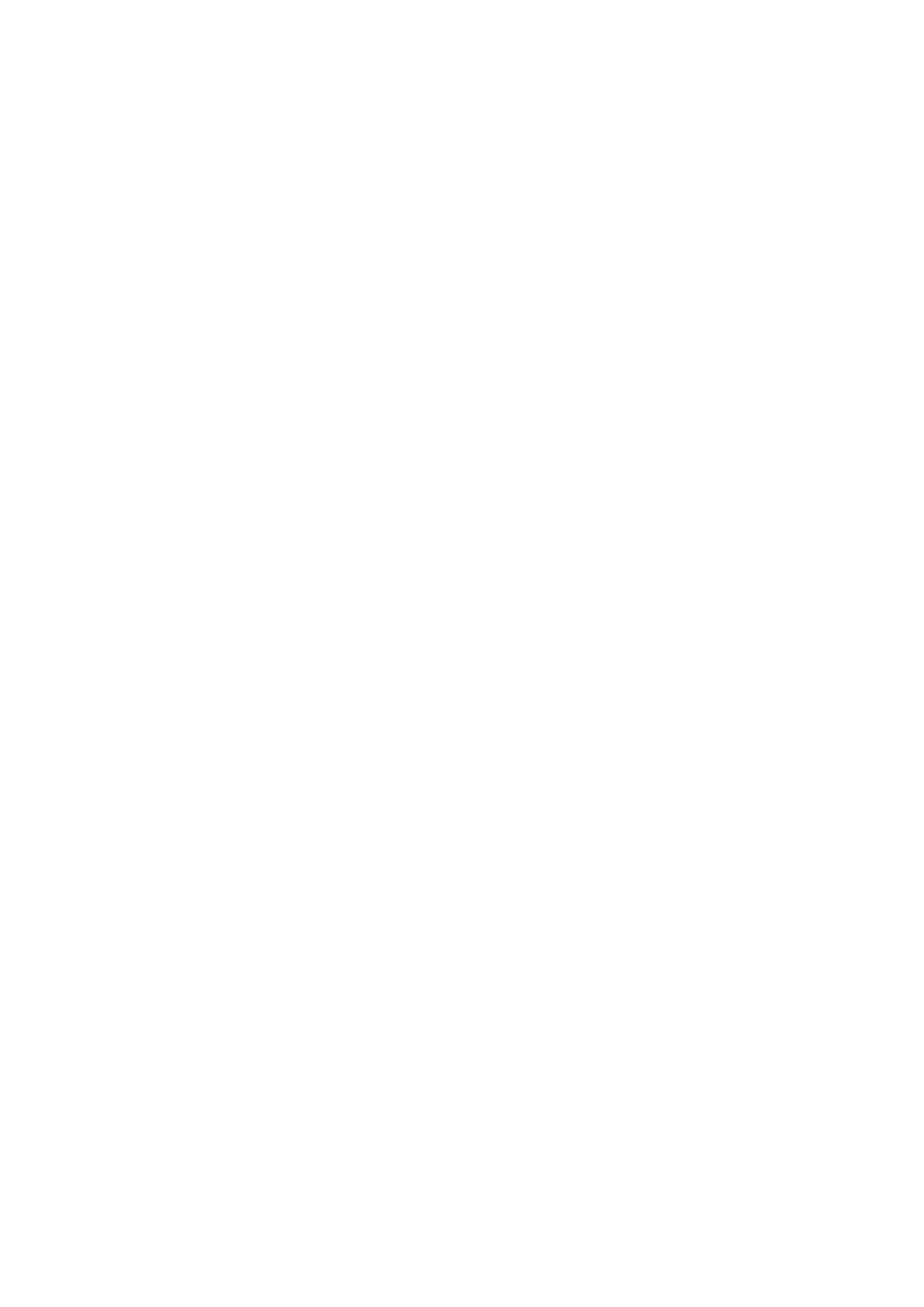
CONSTRAINT FK_LoanPreparer
FOREIGN KEY REFERENCES Employees(EmployeeID),
CustomerID int NOT NULL
CONSTRAINT FK_LoanReceiver
FOREIGN KEY REFERENCES Customers(CustomerID),
AccountNumber char(10),
LoanTypeID int NOT NULL
CONSTRAINT FK_LoanTypes
FOREIGN KEY REFERENCES LoanTypes(LoanTypeID),
LoanAmount money NOT NULL,
InterestRate decimal(6,2) NOT NULL,
Periods decimal(6,2) NOT NULL,
InterestAmount AS
((LoanAmount*(InterestRate/(100)))*(Periods/(12))),
FutureValue AS
(LoanAmount+(LoanAmount*(InterestRate/(100)))*(Periods/(12))),
MonthlyPayment AS
((LoanAmount+(LoanAmount*(InterestRate/(100)))*(Periods/(12)))/Period
s),
Notes Text,
CONSTRAINT PK_LoanAllocations PRIMARY KEY(LoanAllocationID)
)
GO
INSERT INTO LoanAllocations(DatePrepared, EmployeeID,
CustomerID, AccountNumber, LoanTypeID, LoanAmount,
InterestRate, Periods, Notes)
VALUES('2/26/2004', 2, 1, '9171394', 4, 6500.00, 12.65, 36,
'The loan will be delivered by our furniture business partner
Helios Furnian');
GO
INSERT INTO LoanAllocations(DatePrepared, EmployeeID,
CustomerID, AccountNumber, LoanTypeID, LoanAmount,
InterestRate, Periods, Notes)
VALUES('06/22/2007', 2, 2, '8628064', 2, 16500.00, 10.20, 60,
'For this car loan, our partner Arlington Honda will process
and deliver the car.');
GO
INSERT INTO LoanAllocations(DatePrepared, EmployeeID,
CustomerID, AccountNumber, LoanTypeID, LoanAmount,
InterestRate, Periods, Notes)
VALUES('12/3/2006', 1, 3, '8468364', 3, 500.00, 18.65, 48,
'This is a regular credit card.');
GO
INSERT INTO LoanAllocations(DatePrepared, EmployeeID,
CustomerID, AccountNumber, LoanTypeID, LoanAmount,
InterestRate, Periods, Notes)
VALUES('08/02/2006', 3, 4, '2483047', 1, 3500.00, 12.74, 36,
'This is personal/cash loan allocated to a customer who walked
in the store and requested it.');
GO
INSERT INTO LoanAllocations(DatePrepared, EmployeeID,
CustomerID, AccountNumber, LoanTypeID, LoanAmount,
InterestRate, Periods, Notes)
VALUES('10/08/2006', 2, 5, '1311804', 4, 22748.36, 12.28, 60,
'This is a regular car financing loan');
GO
-- =========================================
-- Table: Payments
-- =========================================
USE WattsALoan
GO