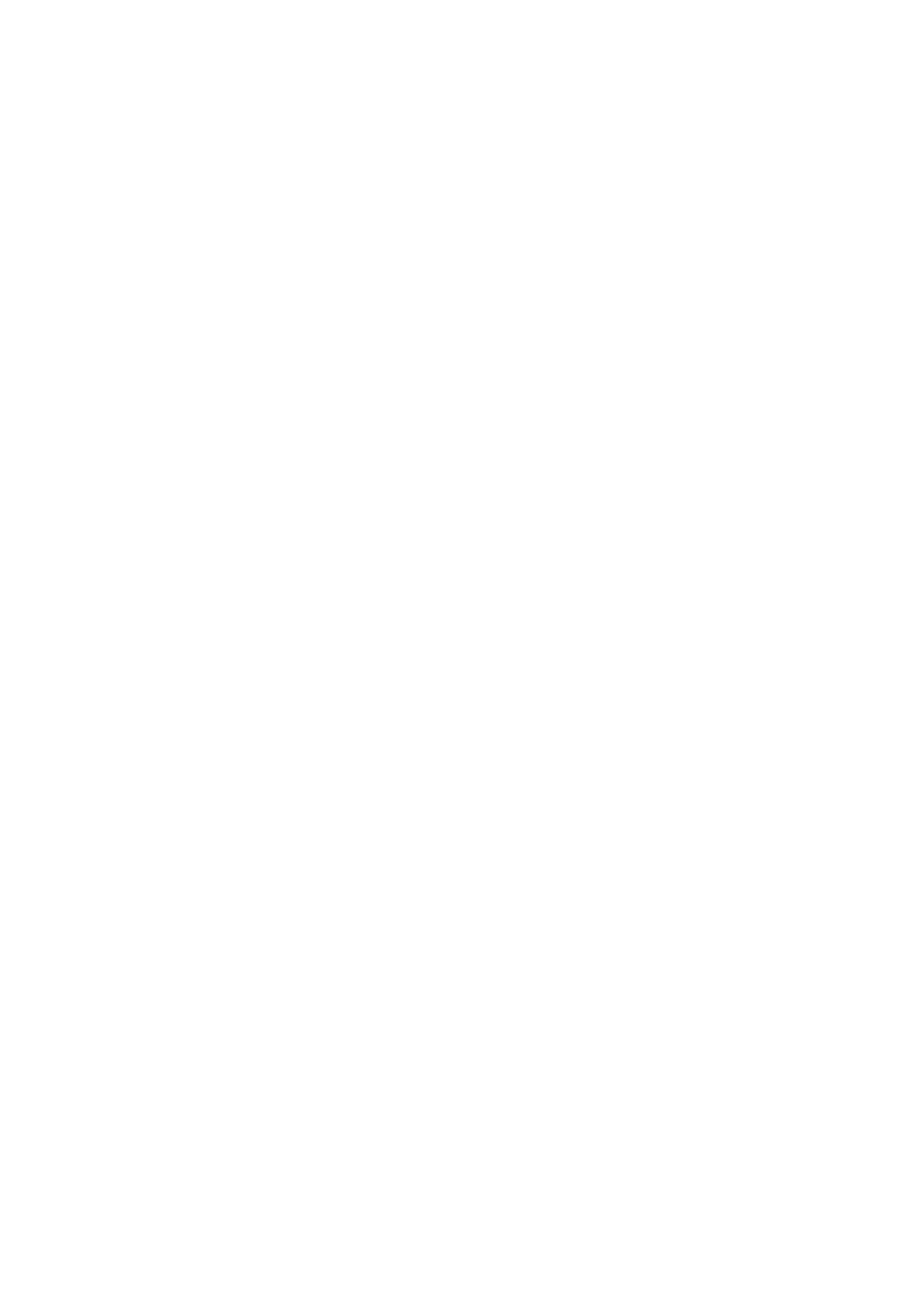
a2 = h
return h
##############
##Class ended##
##############
u = polynome(coeff = [1, 2], x = 1, a = 1, b = 2) #Ici j'appelle la class polynome
print('P(x) = ', u.poly())
print('dP(x) = ', u.derive())
print('integral_P(x) = ', u.integral())
from scipy.integrate import odeint
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 20, 100)
def model(y, x, k):
dy= k*y
return dy
k = 0.1
y0 = odeint(model, 5, x, (k, ))
k = 0.3
y1 = odeint(model, 5, x, (k, ))
k = 0.5
y2 = odeint(model, 5, x, (k, ))
k = 1.2
y3 = odeint(model, 5, x, (k, ))