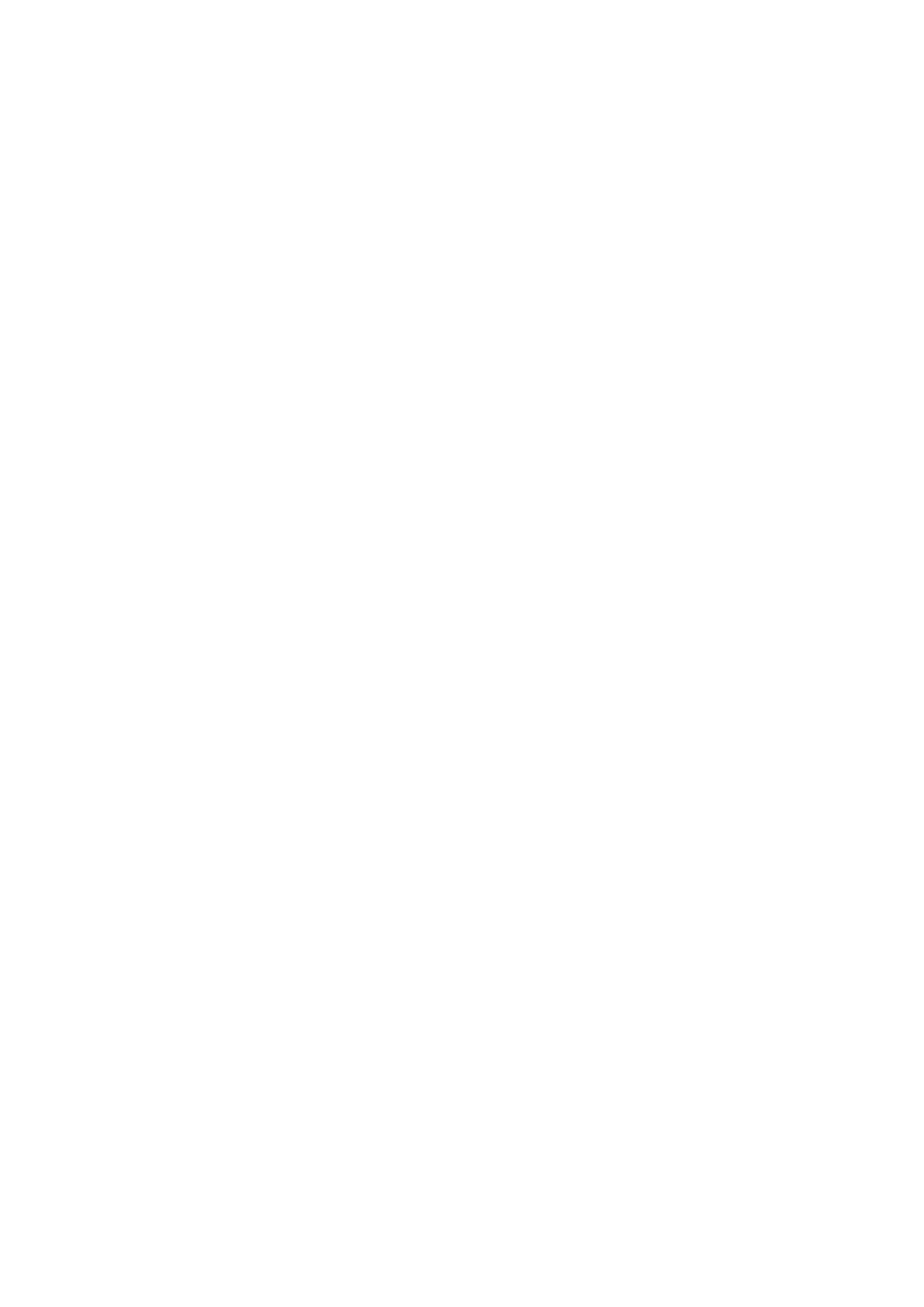
0,c[d],d,a)){b=d;break a}b=-1}return 0>b?null:"string"===typeof
a?a.charAt(b):a[b]}function Ua(a,b){a:if("string"===typeof
a)a="string"!==typeof b||1!=b.length?-1:a.indexOf(b,0);else{for(var
c=0;c<a.length;c++)if(c in a&&a[c]===b){a=c;break a}a=-1}return
0<=a};function Va(a){return
function(){return!a.apply(this,arguments)}}function Wa(a){var
b=!1,c;return function(){b||(c=a(),b=!0);return c}}function Xa(a){var
b=a;return function(){if(b){var c=b;b=null;c()}}};function Ya(a,b){var
c={},d;for(d in a)b.call(void 0,a[d],d,a)&&(c[d]=a[d]);return c}function
Za(a,b){for(var c in a)if(b.call(void
0,a[c],c,a))return!0;return!1}function $a(a,b){return null!==a&&b in
a}function ab(a,b){for(var c in a)if(b.call(void 0,a[c],c,a))return
c}function bb(a){var b={},c;for(c in a)b[c]=a[c];return b};var
cb;function db(){if(void 0===cb){var
a=null,b=x.trustedTypes;if(b&&b.createPolicy){try{a=b.createPolicy("goog#
html",{createHTML:Ma,createScript:Ma,createScriptURL:Ma})}catch(c){x.cons
ole&&x.console.error(c.message)}cb=a}else cb=a}return cb};function
eb(a,b){this.c=a===fb&&b||"";this.f=gb}eb.prototype.b=!0;eb.prototype.a=f
unction(){return this.c};function hb(a){return a instanceof
eb&&a.constructor===eb&&a.f===gb?a.c:"type_error:Const"}var
gb={},fb={};var ib={};function
jb(a,b){this.c=b===ib?a:"";this.b=!0}function kb(a,b){for(var
c=[],d=1;d<arguments.length;d++)c.push(JSON.stringify(arguments[d]).repla
ce(/</g,"\\x3c"));c="("+hb(a)+")("+c.join(",
")+");";c=(d=db())?d.createScript(c):c;return new
jb(c,ib)}jb.prototype.a=function(){return this.c.toString()};function
lb(a){return a instanceof
jb&&a.constructor===jb?a.c:"type_error:SafeScript"};function
mb(a,b){this.c=b===nb?a:""}mb.prototype.b=!0;mb.prototype.a=function(){re
turn this.c.toString()};function ob(a){return a instanceof
mb&&a.constructor===mb?a.c:"type_error:TrustedResourceUrl"}
function pb(){var a={},b=hb(new
eb(fb,"https://pagead2.googlesyndication.com/pagead/gen_204"));if(!qb.tes
t(b))throw Error("Invalid TrustedResourceUrl format: "+b);var
c=b.replace(rb,function(d,e){if(!Object.prototype.hasOwnProperty.call(a,e
))throw Error('Found marker, "'+e+'", in format string, "'+b+'", but no
valid label mapping found in args: '+JSON.stringify(a));d=a[e];return d
instanceof eb?hb(d):encodeURIComponent(String(d))});return sb(c)}
var rb=/%{(\w+)}/g,qb=/^((https:)?\/\/[0-9a-z.:[\]-
]+\/|\/[^/\\]|[^:/\\%]+\/|[^:/\\%]*[?#]|about:blank#)/i,tb=/^([^?#]*)(\?[
^#]*)?(#[\s\S]*)?/;function ub(a){var
b=pb();b=tb.exec(ob(b).toString());var c=b[3]||"";return
sb(b[1]+vb("?",b[2]||"",a)+vb("#",c,void 0))}var nb={};function sb(a){var
b=db();a=b?b.createScriptURL(a):a;return new mb(a,nb)}
function vb(a,b,c){if(null==c)return b;if("string"===typeof c)return
c?a+encodeURIComponent(c):"";for(var d in
c)if(Object.prototype.hasOwnProperty.call(c,d)){var
e=c[d];e=Array.isArray(e)?e:[e];for(var f=0;f<e.length;f++){var
g=e[f];null!=g&&(b||(b=a),b+=(b.length>a.length?"&":"")+encodeURIComponen
t(d)+"="+encodeURIComponent(String(g)))}}return b};function
wb(a){return/^[\s\xa0]*([\s\S]*?)[\s\xa0]*$/.exec(a)[1]}var
xb=/&/g,yb=/</g,zb=/>/g,Ab=/"/g,Bb=/'/g,Cb=/\x00/g;
function Db(a,b){var
c=0;a=wb(String(a)).split(".");b=wb(String(b)).split(".");for(var
d=Math.max(a.length,b.length),e=0;0==c&&e<d;e++){var
f=a[e]||"",g=b[e]||"";do{f=/(\d*)(\D*)(.*)/.exec(f)||["","","",""];g=/(\d
*)(\D*)(.*)/.exec(g)||["","","",""];if(0==f[0].length&&0==g[0].length)bre
ak;c=Eb(0==f[1].length?0:parseInt(f[1],10),0==g[1].length?0:parseInt(g[1]
,10))||Eb(0==f[2].length,0==g[2].length)||Eb(f[2],g[2]);f=f[3];g=g[3]}whi
le(0==c)}return c}function Eb(a,b){return a<b?-1:a>b?1:0};function
Fb(a,b){this.c=b===Gb?a:""}Fb.prototype.b=!0;Fb.prototype.a=function(){re