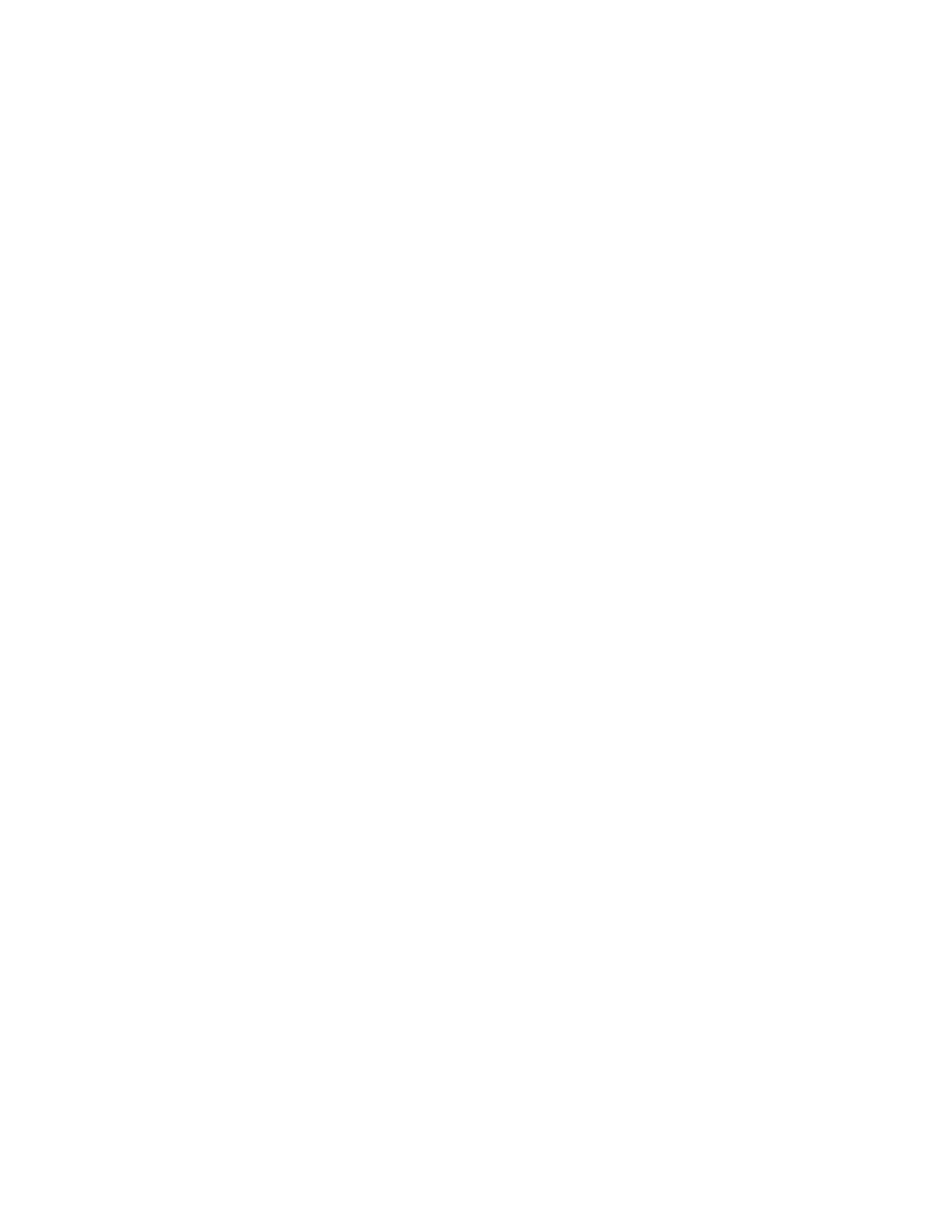
Accelerometer)
!
\%,#!',$$!16!$%,!)00,(,-.5,$,-!5)Q,!'1-,!$.!0%,0Q!$%,!-)#2,!)#/!',#'&$&J&$47!;#!$%,!=:>?@AB!$%,!
/,3)1($!-)#2,!&'!DEFFF!$.!EFFF!/,2R'7!8%&'!0)##.$!*,!5./&3&,/G!*1$!&3!4.1!0)#!0%)#2,!$%,!-)#2,!.#!4.1-!
)00,(,-.5,$,-!4.1!5&2%$!+)#$!$.!/,0-,)',!&$!'.!&$!&'!5.-,!',#'&$&J,!$.!'5)((,-!0%)#2,'!)'!4.1!+&((!(&Q,(4!
#.$!',,!-.$)$&.#!J)(1,'!)'!()-2,!)'!EFFF!/,2R'7!!
"#!.-/,-!$.!.*$)&#!$%,!)#2(,!3-.5!$%,!)00,(,-.5,$,-!/)$)G!$%,-,!)-,!)!3,+!5,$%./'!4.1!0)#!1',7!\,!+&((!
2.!.J,-!$%-,,!5,$%./'!&#!$%&'!()*7!8%,',!5,$%./'!)-,!/&'01'',/!*,(.+7!
!
Small)Angle)Approximation)
!
8%,!'5)((!)#2(,!)66-.K&5)$&.#!5,$%./!1','!$-&2!$.!0)(01()$,!$%,!)#2(,G!*1$!)''15,'!$%)$!'&#b!c!b7!8%&'!&'!
)#!)001-)$,!,'$&5)$,!3.-!)#2(,'!(,''!$%)#!@Fd7!C&J,#!)!#.-5)(!5.$.-!0.#$-.((,-G!&3!$%,!$&($!)#2(,!&'!2-,)$,-!
$%)#!@F!/,2-,,'G!$%,!0.#$-.((,-!+&((!(&Q,(4!#,,/!$.!*,!-1##!)$!31((!'6,,/!$.!0.--,0$!$%&'!$&($!)#/!
6-,0&'&.#!)#2(,!5,)'1-,5,#$!&'!#.$!-,P1&-,/!3.-!$%&'7!8%,-,3.-,!1'!$%,!'5)((!)#2(,!)66-.K&5)$&.#!+&((!
+.-Q!+,((7!8%,!3.-51()!*,(.+!1','!$%&'!)''156$&.#!$.!0)(01()$,!$%,!KD)#2(,!3-.5!$%,!)00,(,-.5,$,-!
-,)/G!$%,!4D)#2(,!&'!0)(01()$,/!$%,!')5,!+)4T!!
K])00!e!N3(.)$ONK])00]=:V!D!K])00].33',$O!f!K])00]'0)(,fMgFR6&h!
\%,-,!$%,!K])00]=:V!&'!$%,!-)+!)00,(,-.5,$,-!-,)/!"$!+)'!3.1#/!1'!)!31#0$&.#!0)((,/!
2,$Z)+:)$)NO!$%)$!0)5,!&#!$%,!/.+#(.)/,/!(&*-)-&,'!3.-!$%&'!)00,(,-.5,$,-7!
8%,!K])00]'0)(,!&'!$%,!0.#J,-'&.#!3)0$.-!$.!0.#J,-$!$%,!?Ua!-,)/!&#$.!2I'7!"$!0)#!*,!3.1#/!&#!$%,!
)00,(,-.5,$,-!/)$)!'%,,$7!!8%,!=:>?!@AB!0.#J,-'&.#!3)0$.-!&'!A52R?Ua!3.-!)((!2!-)#2,'G!$%,-,3.-,!&$I'!
K])00]'0)(,!e!F7FFA7!"#!.-/,-!$.!1',!$%,!$-&2!0)(01()$&.#'!)#/!'5)((!)#2(,!)66-.K&5)$&.#G!$%,!)00,(,-)$&.#!
8%,!.33',$!J)(1,!&'!$%,!J)(1,!$%,!)00,(,-.5,$,-!-,)/'!+%,#!&$!&'!'$)$&.#)-4!)#/!(4!3()$7!"$!+)'!.*$)&#,/!
*4!)J,-)2!$%,!-,)/I'!.3!$%,!)#2(,!+%,#!$%,!)00,(,-.5,$,-!+)'!(4!3()$!3.-!E!',0.#/'7!!
8%,!MgFR6&!0.#J,-$'!$%,!2!J)(1,!&#$.!)#!)#2(,!&#!/,2-,,'7!!
8%&'!5,$%./!2&J,'!)!3)&-(4!)001-)$,!-,)/!3.-!$%,!)#2(,7!W.+,J,-G!*,0)1',!&$!.#(4!1','!.#,!)K&'!$.!
0)(01()$,!$%,!)#2(,!&$!+&((!2&J,!)#!)#2(,!*,$+,,#!D^F!)#/!^F!/,2-,,'7!8%&'!'%.1(/!*,!3&#,!3.-!)!*)()#0!
)66(&0)$&.#!*,0)1',!&3!4.1!)-,!.33!*4!5.-,!$%)#!^F!/,2-,,'!$%)#!4.1!(&Q,(4!+.#I$!*,!)*(,!$.!0.--,0$!&$!
)#4+)47!"3!)#!)#2(,!*,$+,,#!D@9F!)#/!@9F!/,2-,,'!&'!-,P1&-,/G!$%,#!$%,!)00,(,-.5,$,-!-,)/!3-.5!
$+.!)K,'!51'$!*,!1',/!$.!0)(01()$,!$%,!)#2(,7!!