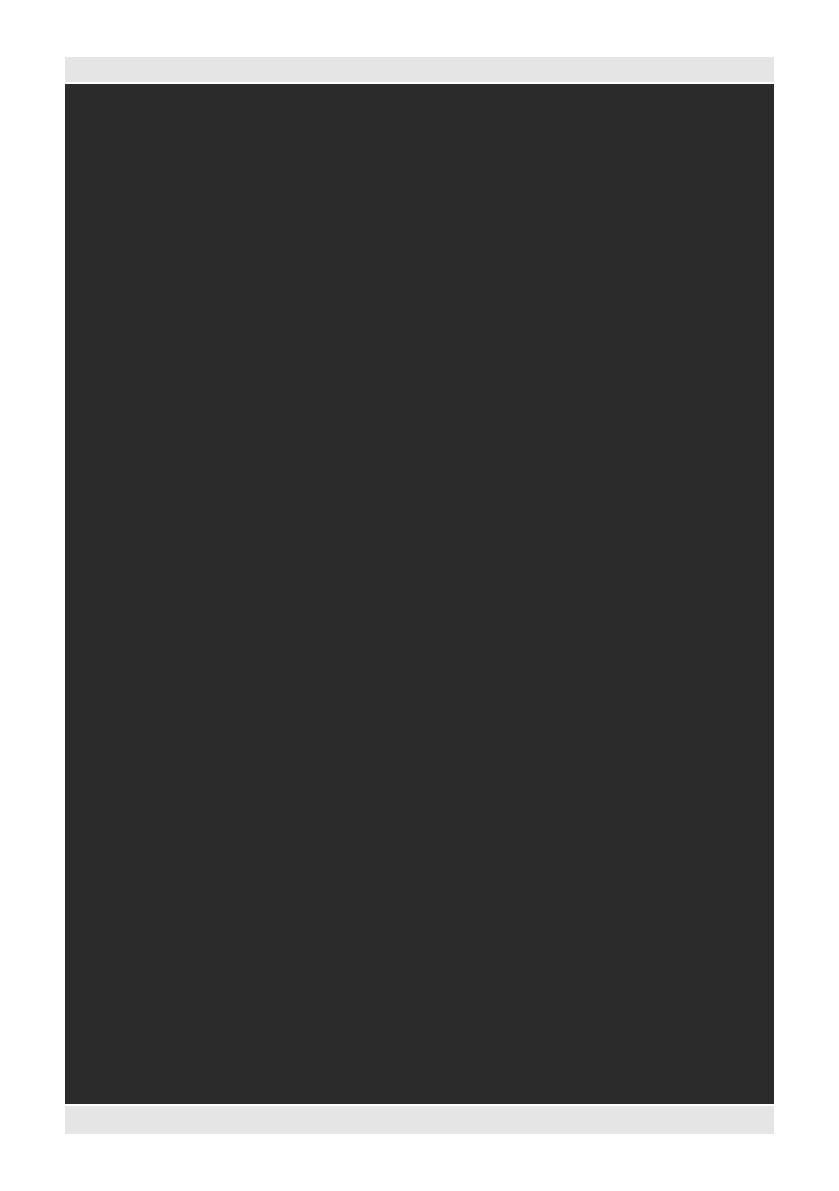
J.D.B. DIASOLUKA Nz. Luyalu
JavaScript Tome-VIII
Miscellaneous - 2 / 19 - vendredi, 31. mai 2019 (7:52 )
const idxc = Symbol('qcmC');
console.log(idxc); // Symbol(qcmC)
const a = [68, 80, 55];
console.log(a, ` , a.length = ${a.length}`);
// Array(3) [ 68, 80, 55 ] , a.length = 3
a[idxv]= 71;
console.log(a, ` , a.length = ${a.length}`);
// Array(3) [ 68, 80, 55 ] , a.length = 3
console.log(`a[idxv] = `, a[idxv]);
// a[idxv] = 71
a[idxv]= 42; // Écrasement de a[idxv]
console.log(a, ` , a.length = ${a.length}`);
// Array(3) [ 68, 80, 55 ] , a.length = 3
console.log(`a[idxv] = `, a[idxv]);
// a[idxv] = 42
a[idxc]= 29;
console.log(a, ` , a.length = ${a.length}`);
// Array(3) [ 68, 80, 55 ] , a.length = 3
console.log(`a[idxc] = `, a[idxc]);
// a[idxc] = 29
a[idxc]= 46; // Écrasement de a[idxc]
console.log(a, ` , a.length = ${a.length}`);
// Array(3) [ 68, 80, 55 ] , a.length = 3
console.log(`a[idxc] = `, a[idxc]);
// a[idxc] = 46
a[a.length]= 95;
console.log(a, ` , a.length = ${a.length}`);
// Array(4) [ 68, 80, 55, 95 ] , a.length = 4
console.log(`a[a.length] = `, a[a.length]);