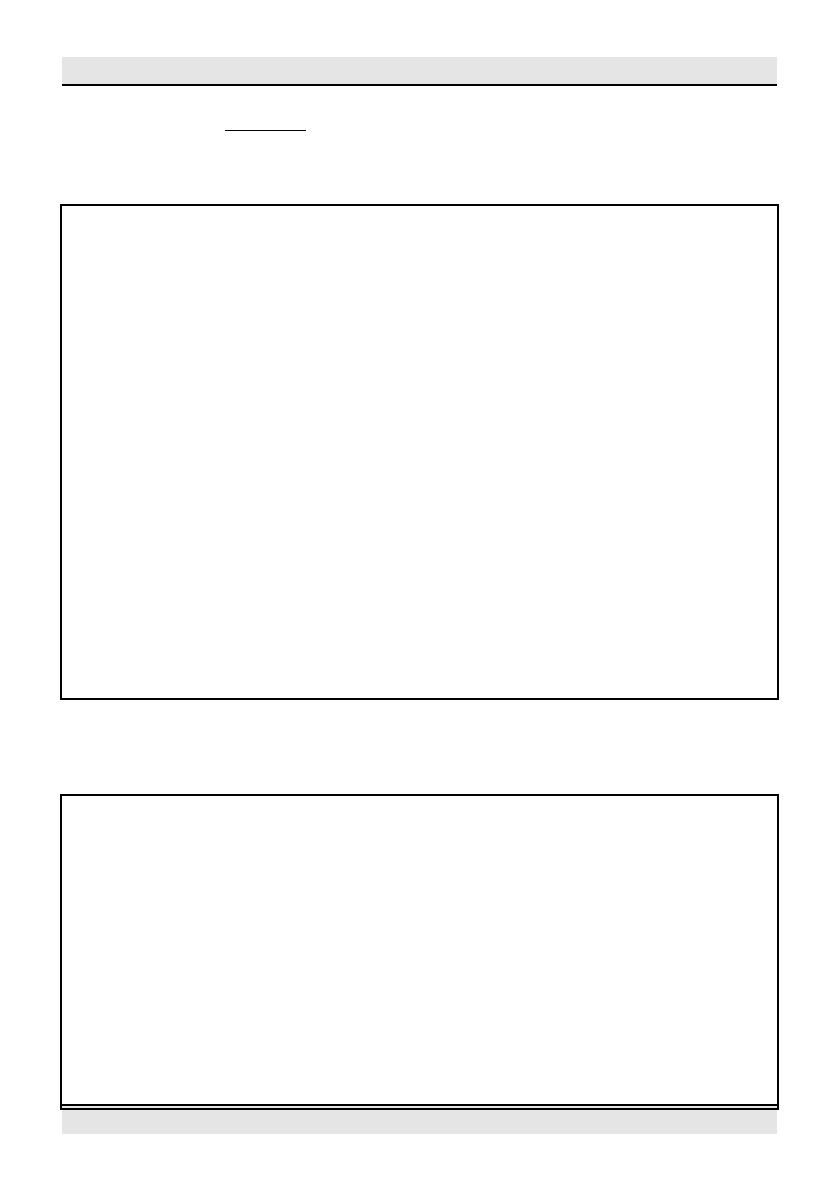
J.D.B. DIASOLUKA Nz.
Luyalu
JavaScript Tome-V
La variable « this »
2 / 96 mardi, 16. octobre 2018
II. En mode STANDARD, « this » dans une fonction représente l’ob-
jet global window. Mais pendant la définition des propriétés
d’une fonction, « this » représente le constructeur et, dans une
instance, il représente l’instance.
<script type="text/javascript">
// En MODE STANDARD,
// this dans une fonction ordinaire
// représente l'objet global widonw.
function o(p){
this.prop=45;
console.log('"',this,'"');
console.log(this===window);
console.log('"',p,'"');
}
o();
/*
" Window " test.html:7:5
true test.html:8:5
" undefined " test.html:9:5
*/
let io=new o(this);
/*
" Object { prop: 45 } " test.html:7:5
false test.html:8:5
" Window ... " test.html:9:5
*/
</script>
III. Dans une instance, « this » représente TOUJOURS l’instance :
<script type="text/javascript"> "use strict";
// this dans une instanc représente
// TOUJOURS un poineur sur l'instance.
function o(p){
this.prop=45;
}
let io=new o();
console.log(io.prop)
let fdummy=p=>p.prop=100;
// fdummy modifie la valeur de prop
// de l'objet lui envoyé.
io.df=function(){fdummy(this)};
// Définition d'une méthode de io qui